用c++编写程序实现以下功能 1. 删除注释 2. 删除续行符以及后续换行符 3. 将换行符和TAB统一替换为空格 4. 将大写字母变换为小写字母,或者相反,以实现不区分大小写 5. 识别标号区,识别续行标志。
时间: 2024-09-19 15:11:47 浏览: 10
在C++中实现上述功能需要结合字符串处理和正则表达式。下面是一个简化的示例,展示了如何分别完成这五个任务:
1. **删除注释** (假设是单行或多行的C风格注释 `//` 或 `/* ... */`)
```cpp
#include <iostream>
#include <string>
using namespace std;
bool isCommentStart(const string& line, size_t pos) {
return pos == 0 || (line[pos - 1] != '/' && line.substr(pos, 2) == "/*");
}
std::string removeComments(string input) {
string output;
size_t start = 0;
for (size_t i = 0; i < input.length(); ++i) {
if (!isCommentStart(input, i)) {
if (start != i) { // 已经跳过注释部分
output += input.substr(start, i - start);
}
if (input[i] == '\n') { // 结束当前行
output += '\n';
}
start = i + 1;
}
}
return output;
}
```
2. **删除续行符和后续换行符**
```cpp
std::string trimContinuationChars(string input) {
return input.replace(input.find_last_of('\r\n'), 1, "");
}
```
3. **替换换行符和TAB**
```cpp
std::string replaceNewlinesAndTabs(std::string input) {
return input.replace(input.find_first_of("\r\n\t"), 1, " ");
}
```
4. **转换大小写**
```cpp
std::string toggleCase(std::string input) {
return std::transform(input.begin(), input.end(), input.begin(), ::tolower); // 变为小写
// 如果需要变大写,可以改为 std::transform(input.begin(), input.end(), input.begin(), ::toupper);
}
```
5. **识别标号区和续行标志**
这个任务通常涉及到更复杂的解析规则,比如C/C++的标识符和关键字。为了简化,这里你可以创建一个函数来判断是否是合法的标识符,并记录续行标志(如`#`):
```cpp
bool isIdentifier(const std::string& token);
bool isContinuationMark(const std::string& line);
std::pair<bool, std::string> processLine(const std::string& line) {
bool isContinued = false;
std::string processedLine;
// 分割并处理每部分
// ... (根据实际规则实现)
return {isContinued, processedLine};
}
```
每个任务单独处理后,你需要在主函数中依次调用它们,将结果组合成最终的处理后的文本。
相关推荐
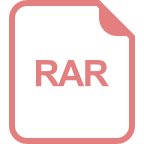
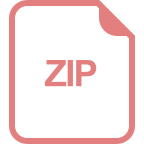
















