springboot security ldap
时间: 2023-12-20 11:01:50 浏览: 154
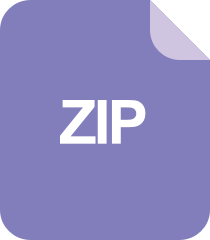
springboot-ldap:该项目的目标是创建一个简单的Spring-Boot REST API(称为简单服务),并使用Spring Security LDAP模块对其进行保护。
Spring Boot Security LDAP是一种基于Spring Boot框架和LDAP(轻型目录访问协议)的安全认证解决方案。LDAP是一种用于访问分布式目录服务的协议,通常用于存储用户和组织的信息。Spring Boot Security LDAP通过整合Spring Security和LDAP,提供了一种简单高效的身份验证和授权机制。
使用Spring Boot Security LDAP,可以将用户信息存储在LDAP目录中,然后通过Spring Security来进行身份认证和授权管理。这样做的好处是可以方便地管理用户和组织的信息,并且在应用中实现统一的认证机制。另外,Spring Boot Security LDAP还提供了灵活的配置选项,可以根据项目的需求进行定制和扩展。
在实际应用中,首先需要配置LDAP服务器的连接信息,如服务器地址、端口号、账号密码等。然后配置Spring Security的LDAP认证提供者,指定LDAP目录中的用户和组织结构。最后,在应用程序中可以使用Spring Security的注解和API来进行权限控制和访问控制。
总的来说,Spring Boot Security LDAP是一种方便、安全和灵活的身份认证解决方案,适用于需要使用LDAP作为用户认证存储的项目。通过整合Spring Boot和LDAP,可以快速构建安全可靠的应用程序,保护用户信息和资源的安全。
阅读全文
相关推荐
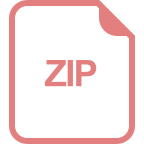
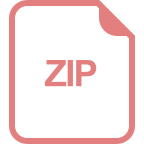





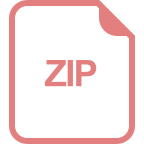
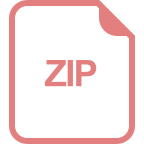






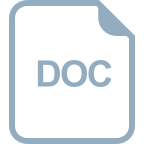
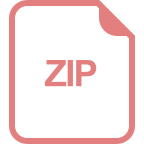