用C语言采用尾插法创建联系人链表
时间: 2024-12-20 14:08:34 浏览: 7
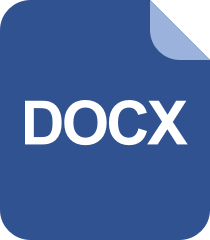
带头结点尾插法创建链表.docx
尾插法是一种常用的链表创建方法,特别适用于创建单链表。使用尾插法创建链表时,我们始终保持一个指向链表末尾的指针,这样可以避免每次插入新节点时都需要从头遍历链表,从而提高效率。下面是用C语言采用尾插法创建联系人链表的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义联系人结构体
typedef struct Contact {
char name[50];
char phone[20];
struct Contact *next;
} Contact;
// 创建联系人链表
Contact* createContactList() {
Contact *head = NULL; // 链表头指针
Contact *tail = NULL; // 链表尾指针
int n;
printf("请输入要创建的联系人数量: ");
scanf("%d", &n);
for (int i = 0; i < n; i++) {
Contact *newContact = (Contact*)malloc(sizeof(Contact));
if (newContact == NULL) {
printf("内存分配失败\n");
exit(1);
}
printf("请输入第%d个联系人的姓名: ", i + 1);
scanf("%s", newContact->name);
printf("请输入第%d个联系人的电话号码: ", i + 1);
scanf("%s", newContact->phone);
newContact->next = NULL;
if (head == NULL) {
head = newContact;
tail = newContact;
} else {
tail->next = newContact;
tail = newContact;
}
}
return head;
}
// 打印联系人链表
void printContactList(Contact *head) {
Contact *current = head;
while (current != NULL) {
printf("姓名: %s, 电话: %s\n", current->name, current->phone);
current = current->next;
}
}
// 主函数
int main() {
Contact *contactList = createContactList();
printf("\n联系人列表:\n");
printContactList(contactList);
return 0;
}
```
这段代码首先定义了一个`Contact`结构体来表示联系人,然后实现了`createContactList`函数来创建联系人链表,`printContactList`函数来打印联系人链表。在`main`函数中,我们调用`createContactList`函数创建联系人链表,并调用`printContactList`函数打印链表内容。
阅读全文
相关推荐
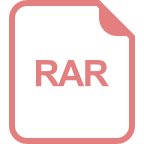
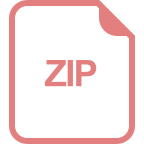
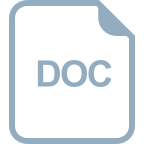
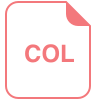
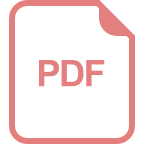
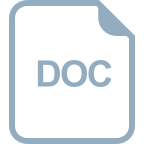
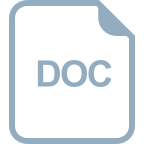
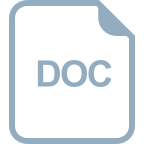


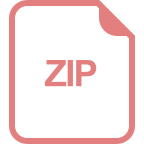
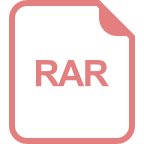