关于sm9的实际演示代码
时间: 2024-12-24 07:06:59 浏览: 3
SM9是一种安全多方计算协议,它主要用于在不暴露原始数据的情况下进行多方计算,比如共享加法、乘法等操作。由于涉及安全性,具体的SM9实际演示代码通常包含一些加密库的调用,例如在中国使用的EasySM或Tencent的secp256k1库。
下面是一个简化版的Python示例,展示了如何使用EasySM库进行SM9的基本运算:
```python
from easysm import SM9
# 初始化SM9密钥对
sk, pk = SM9.generate_key_pair()
# 对两个隐匿的数据进行加法
data1, data2 = "隐藏数据1", "隐藏数据2"
shared_secret = sk.add(pk, data1.encode(), data2.encode())
# 公开的秘钥可以验证结果
public_key = sm9.get_public_key()
if shared_secret.verify(public_key, data1.encode() + data2.encode()):
print("加法运算成功")
else:
print("加法运算失败")
相关问题
java sm2 代码
以下是一个简单的Java代码示例,用于生成SM2公私钥对并进行加密解密操作:
```java
import org.bouncycastle.crypto.AsymmetricCipherKeyPair;
import org.bouncycastle.crypto.CipherParameters;
import org.bouncycastle.crypto.InvalidCipherTextException;
import org.bouncycastle.crypto.engines.SM2Engine;
import org.bouncycastle.crypto.generators.AsymmetricCipherKeyPairGenerator;
import org.bouncycastle.crypto.generators.ECKeyPairGenerator;
import org.bouncycastle.crypto.params.*;
import org.bouncycastle.crypto.signers.SM2Signer;
import org.bouncycastle.math.ec.ECPoint;
import java.math.BigInteger;
import java.security.SecureRandom;
public class SM2Example {
public static void main(String[] args) throws InvalidCipherTextException {
// 生成SM2密钥对
AsymmetricCipherKeyPair keyPair = generateSM2KeyPair();
// 获取公私钥参数
ECPrivateKeyParameters privateKey = (ECPrivateKeyParameters) keyPair.getPrivate();
ECPublicKeyParameters publicKey = (ECPublicKeyParameters) keyPair.getPublic();
// 要加密的明文
byte[] plaintext = "Hello, SM2!".getBytes();
// 使用公钥进行加密
byte[] ciphertext = encryptWithSM2(publicKey, plaintext);
System.out.println("Ciphertext: " + new String(ciphertext));
// 使用私钥进行解密
byte[] decryptedText = decryptWithSM2(privateKey, ciphertext);
System.out.println("Decrypted Text: " + new String(decryptedText));
// 签名和验签示例
byte[] message = "Message to be signed".getBytes();
byte[] signature = signWithSM2(privateKey, message);
boolean isVerified = verifyWithSM2(publicKey, message, signature);
System.out.println("Signature Verification: " + isVerified);
}
// 生成SM2公私钥对
public static AsymmetricCipherKeyPair generateSM2KeyPair() {
AsymmetricCipherKeyPairGenerator generator = new ECKeyPairGenerator();
generator.init(new ECKeyGenerationParameters(SM2Util.getDomainParameters(), new SecureRandom()));
return generator.generateKeyPair();
}
// 使用公钥进行加密
public static byte[] encryptWithSM2(ECPublicKeyParameters publicKey, byte[] plaintext) throws InvalidCipherTextException {
SM2Engine engine = new SM2Engine();
engine.init(true, new ParametersWithRandom(publicKey, new SecureRandom()));
return engine.processBlock(plaintext, 0, plaintext.length);
}
// 使用私钥进行解密
public static byte[] decryptWithSM2(ECPrivateKeyParameters privateKey, byte[] ciphertext) throws InvalidCipherTextException {
SM2Engine engine = new SM2Engine();
engine.init(false, privateKey);
return engine.processBlock(ciphertext, 0, ciphertext.length);
}
// 使用私钥进行签名
public static byte[] signWithSM2(ECPrivateKeyParameters privateKey, byte[] message) {
SM2Signer signer = new SM2Signer();
signer.init(true, privateKey);
signer.update(message, 0, message.length);
return signer.generateSignature();
}
// 使用公钥进行验签
public static boolean verifyWithSM2(ECPublicKeyParameters publicKey, byte[] message, byte[] signature) {
SM2Signer verifier = new SM2Signer();
verifier.init(false, publicKey);
verifier.update(message, 0, message.length);
return verifier.verifySignature(signature);
}
}
```
在上面的示例中,我们使用了Bouncy Castle密码库(Bouncy Castle Crypto Library)来实现SM2加密算法。你需要确保已经将Bouncy Castle库添加到项目的依赖中。
此示例演示了如何生成SM2公私钥对、使用公钥进行加密、使用私钥进行解密,以及使用私钥进行签名和使用公钥进行验签。请注意,示例中的加密和解密操作是基于SM2原生的非对称加密算法,而签名和验签操作是基于SM2的数字签名算法。
你可以根据自己的需求对代码进行进一步的扩展和优化。同时,建议在实际使用时,对密钥进行适当的存储和保护,确保安全性。
SM4的CTR模式,使用C语言不借助库实现,代码示例
SM4(Stream Cipher Mode 4)是一种密码块加密标准,用于对数据流进行加密。CTR(Counter)模式是一种常用的密钥流生成方式,它通过将明文长度与密钥相结合,生成一个唯一的加密计数器(Counter),然后与密钥进行异或操作得到密钥流。
以下是使用C语言不借助外部库实现SM4 CTR模式的一个简单示例:
```c
#include <stdint.h>
// SM4 key (32 bytes) and initialization vector (IV, also 32 bytes)
uint8_t sm4_key[32], iv[32];
// SM4 S-boxes and other constants
const uint8_t sbox[256][4] = ...; // 实现SM4 S-box的具体数组
const uint32_t rcon[] = ...; // Rcon序列
// Function to perform AES-like SubBytes operation (SM4 S-box)
void sub_bytes(uint8_t* input) {
int i;
for (i = 0; i < 4; ++i) {
input[i] = sbox[input[i]];
}
}
// Function to perform the SM4 CTR encryption
uint8_t sm4_ctr_encrypt(const uint8_t* plaintext, size_t len) {
uint8_t keystream[16], counter[4];
size_t pos;
// Load IV into counter array
memcpy(counter, iv, sizeof(counter));
// XOR plaintext with keystream
for (pos = 0; pos < len; pos += 16) {
// Generate next keystream block
uint32_t keystream_word;
for (int i = 0; i < 4; ++i) {
keystream_word |= ((counter[i] << (8 * (3 - i))) ^ plaintext[pos + i]) & 0xFF;
}
// Apply SubBytes and MixColumns transformations
sub_bytes((uint8_t*)&keystream_word);
for (int j = 0; j < 4; ++j) {
keystream_word = mix_columns(keystream_word, j);
}
// Update counter
counter[3]++;
if (counter[3] == 0) {
counter[2]++; // Roll over after reaching FF
if (counter[2] == 0) {
counter[1]++;
if (counter[1] == 0) {
counter[0]++;
if (counter[0] == 0 && rcon[0]) { // Use first Rcon on IV if needed
sub_bytes(counter);
for (int i = 0; i < 4; ++i) {
keystream_word ^= rcon[0] << (8 * i);
}
}
}
}
}
// XOR plaintext with keystream
for (int i = 0; i < 16; ++i) {
plaintext[pos + i] ^= keystream[i];
}
}
return plaintext;
}
```
注意:此代码仅作教学演示,实际应用中需要确保所有S-box、MixColumns和其他密钥流生成细节都准确无误地实现了SM4规范。由于SM4相对复杂,这里省略了许多细节,如轮函数的实现、循环移位等。此外,真正的实现通常会考虑性能优化和安全性考虑,比如内联S-box运算、缓存友好的内存访问等。
阅读全文
相关推荐
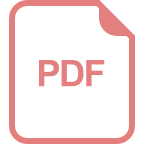
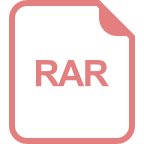
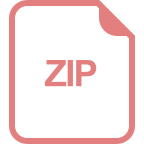
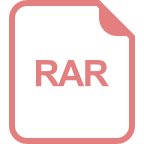
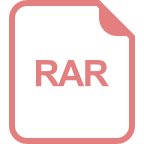
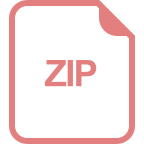
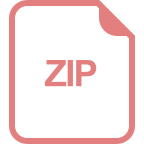
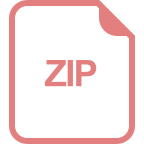
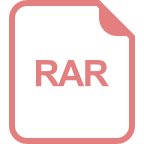
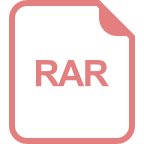
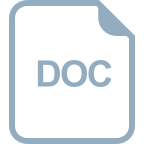
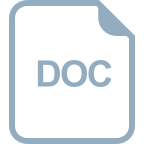
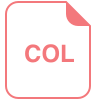
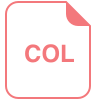
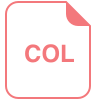
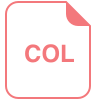
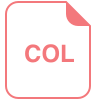