C#winform的DataGridView控件的文本列若我不想设置值,使其显示空白该如何做
时间: 2024-12-09 11:28:47 浏览: 18
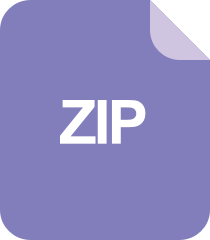
C# winform分页控件,用于dataGridView分页浏览,分页,跳转,自定义页数,动态设置页大小
在C# WinForms中,如果你希望DataGridView控件的文本列显示空白而不是默认值或空字符串,可以通过以下几种方式实现:
### 方法一:设置单元格的默认值为null
你可以在绑定数据源之前,将DataGridView的单元格默认值设置为`DBNull.Value`。这样,当没有数据时,单元格会显示为空白。
```csharp
dataGridView1.Columns["YourColumnName"].DefaultCellStyle.NullValue = DBNull.Value;
```
### 方法二:处理DataBinding事件
你可以订阅DataGridView的`DataBindingComplete`事件,并在事件处理程序中检查并设置单元格的值为空白。
```csharp
private void dataGridView1_DataBindingComplete(object sender, DataGridViewBindingCompleteEventArgs e)
{
foreach (DataGridViewRow row in dataGridView1.Rows)
{
if (row.Cells["YourColumnName"].Value == null || row.Cells["YourColumnName"].Value == DBNull.Value)
{
row.Cells["YourColumnName"].Value = string.Empty;
}
}
}
```
### 方法三:自定义单元格格式
你可以通过设置DataGridView的`CellFormatting`事件来自定义单元格的显示内容。
```csharp
private void dataGridView1_CellFormatting(object sender, DataGridViewCellFormattingEventArgs e)
{
if (e.ColumnIndex == dataGridView1.Columns["YourColumnName"].Index && e.Value == null)
{
e.Value = string.Empty; // 或者使用空格 " "
e.FormattingApplied = true;
}
}
```
### 方法四:在数据源中设置默认值
如果你有控制权,可以在数据源中设置默认值为空字符串或null,这样在绑定到DataGridView时会自动显示为空白。
```csharp
// 假设你有一个DataTable作为数据源
DataTable table = new DataTable();
table.Columns.Add("YourColumnName", typeof(string));
table.Rows.Add(DBNull.Value); // 添加一个空值行
dataGridView1.DataSource = table;
```
### 示例代码
以下是一个完整的示例代码,展示了如何通过上述方法之一来实现DataGridView文本列显示空白:
```csharp
using System;
using System.Data;
using System.Windows.Forms;
public class MainForm : Form
{
private DataGridView dataGridView1;
public MainForm()
{
dataGridView1 = new DataGridView();
dataGridView1.Dock = DockStyle.Fill;
this.Controls.Add(dataGridView1);
// 创建示例数据表
DataTable table = new DataTable();
table.Columns.Add("YourColumnName", typeof(string));
table.Rows.Add(DBNull.Value); // 添加一个空值行
dataGridView1.DataSource = table;
// 订阅DataBindingComplete事件
dataGridView1.DataBindingComplete += dataGridView1_DataBindingComplete;
}
private void dataGridView1_DataBindingComplete(object sender, DataGridViewBindingCompleteEventArgs e)
{
foreach (DataGridViewRow row in dataGridView1.Rows)
{
if (row.Cells["YourColumnName"].Value == null || row.Cells["YourColumnName"].Value == DBNull.Value)
{
row.Cells["YourColumnName"].Value = string.Empty;
}
}
}
[STAThread]
public static void Main()
{
Application.EnableVisualStyles();
Application.Run(new MainForm());
}
}
```
以上代码展示了如何在WinForms应用程序中使用DataGridView控件,并通过多种方式确保文本列在没有数据时显示为空白。
阅读全文
相关推荐
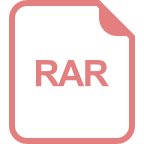
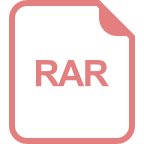
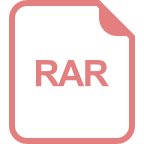
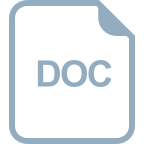
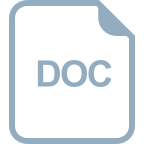












