C# 每秒给RabbitMQ发送一次消息
时间: 2024-09-12 19:15:33 浏览: 45
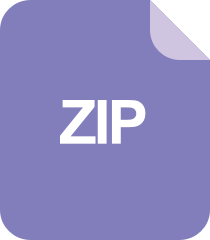
C# .Net RabbitMQ消息队列发送和接收实例程序

在C#中,要实现每秒向RabbitMQ发送一次消息,你可以使用RabbitMQ的.NET客户端库,例如RabbitMQ.Client。以下是一个简单的实现步骤:
1. 安装RabbitMQ.Client库:你可以通过NuGet包管理器来安装它,使用命令`Install-Package RabbitMQ.Client`。
2. 创建消息生产者:首先,你需要创建一个`ConnectionFactory`对象,然后通过它创建`IConnection`和`IModel`对象。`IModel`对象代表了与RabbitMQ服务器的通道。
3. 声明交换器和队列:在发送消息之前,你需要声明一个交换器(Exchange)和队列(Queue),并设置它们之间的绑定关系。
4. 设置定时器:使用`System.Timers.Timer`类来创建一个定时器,设置其`Interval`属性为1000毫秒(即1秒),并设置`AutoReset`为true。然后在定时器的`Elapsed`事件中编写发送消息的逻辑。
5. 发送消息:在定时器事件的回调函数中,使用`IModel`对象的`basicPublish`方法来发送消息。
下面是一个简单的示例代码:
```csharp
using RabbitMQ.Client;
using System;
using System.Timers;
class Program
{
static void Main(string[] args)
{
var factory = new ConnectionFactory() { HostName = "localhost" };
using (var connection = factory.CreateConnection())
using (var channel = connection.CreateModel())
{
channel.QueueDeclare(queue: "hello",
durable: false,
exclusive: false,
autoDelete: false,
arguments: null);
var properties = channel.CreateBasicProperties();
properties.Persistent = true; // 持久化消息
var timer = new Timer();
timer.Interval = 1000; // 设置时间间隔为1秒
timer.Elapsed += (sender, e) =>
{
string message = "Hello World!";
var body = System.Text.Encoding.UTF8.GetBytes(message);
channel.BasicPublish(exchange: "",
routingKey: "hello",
basicProperties: properties,
body: body);
};
timer.AutoReset = true;
timer.Enabled = true;
Console.WriteLine(" Press [enter] to exit.");
Console.ReadLine();
}
}
}
```
确保你的RabbitMQ服务正在运行,而且你已经正确地设置了队列和交换器的名称。
阅读全文
相关推荐
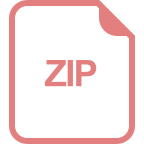
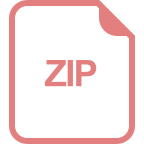
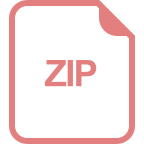
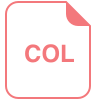
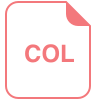
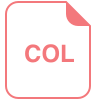
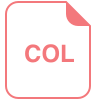
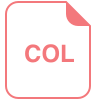
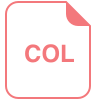
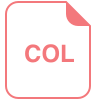
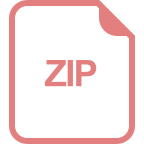
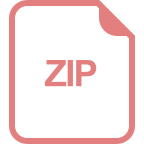