在c#桌面应用中对数据进行Ms2加密
时间: 2024-11-24 22:42:49 浏览: 3
在C#的桌面应用程序中,对数据进行MS2加密通常是指使用Microsoft Security.Cryptography套件提供的加密算法。MS2并不是一个特定的加密标准,可能是误拼或者指的是某种自定义加密方法。但如果是想指代Windows平台下的强加密,可以考虑使用如AES(Advanced Encryption Standard)或其他FIPS认证的算法。
例如,你可以使用`System.Security.Cryptography.Aes`类来进行AES加密操作。下面是一个基本的示例,展示如何创建一个AES密钥和使用它来加密和解密字符串:
```csharp
using System;
using System.IO;
using System.Security.Cryptography;
public class AESExample
{
private static readonly byte[] Salt = new byte[] { /* Your salt value here */ };
public static string Encrypt(string plainText)
{
using (Aes aes = Aes.Create())
{
// 使用一个随机生成的初始向量
aes.IV = GenerateIV();
// 设置Key从用户密码派生
Rfc2898DeriveBytes keyDerivation = new Rfc2898DeriveBytes("YourPassword", Salt);
aes.Key = keyDerivation.GetBytes(aes.KeySize / 8);
ICryptoTransform encryptor = aes.CreateEncryptor(aes.Key, aes.IV);
// 创建一个内存流用于加密
using (MemoryStream msEncrypt = new MemoryStream())
{
using (CryptoStream csEncrypt = new CryptoStream(msEncrypt, encryptor, CryptoStreamMode.Write))
{
using (StreamWriter swEncrypt = new StreamWriter(csEncrypt))
{
swEncrypt.Write(plainText);
}
return Convert.ToBase64String(msEncrypt.ToArray());
}
}
}
}
public static string Decrypt(string encryptedText)
{
try
{
byte[] encryptedData = Convert.FromBase64String(encryptedText);
using (Aes aes = Aes.Create())
{
aes.IV = Convert.FromBase64String(encryptedText.Substring(0, aes.BlockSize)); // Assuming the IV is at the start of the base64 string
// 使用相同的盐和密码来解密
Rfc2898DeriveBytes keyDerivation = new Rfc2898DeriveBytes("YourPassword", Salt);
aes.Key = keyDerivation.GetBytes(aes.KeySize / 8);
ICryptoTransform decryptor = aes.CreateDecryptor(aes.Key, aes.IV);
using (MemoryStream msDecrypt = new MemoryStream(encryptedData))
{
using (CryptoStream csDecrypt = new CryptoStream(msDecrypt, decryptor, CryptoStreamMode.Read))
{
using (StreamReader srDecrypt = new StreamReader(csDecrypt))
{
return srDecrypt.ReadToEnd();
}
}
}
}
}
catch (Exception ex)
{
Console.WriteLine($"Error: {ex.Message}");
return null;
}
}
private static byte[] GenerateIV()
{
using (Aes aes = Aes.Create())
{
return aes.IV;
}
}
}
// 示例用法
string plaintext = "Your data to be encrypted";
string encrypted = AESExample.Encrypt(plaintext);
string decrypted = AESExample.Decrypt(encrypted);
阅读全文
相关推荐
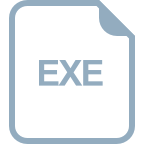
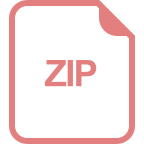















