线性表的顺序存储代码
时间: 2024-09-14 15:00:45 浏览: 39
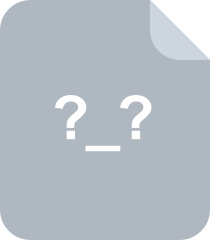
线性表的顺序存储C语言代码
线性表的顺序存储是指使用一段连续的存储单元来存储线性表的数据元素,这种存储结构通常称为数组。在线性表的顺序存储结构中,数据元素之间的逻辑关系是通过元素之间的相对位置来表示的。
以下是一个简单的线性表顺序存储的实现代码,使用Python语言为例:
```python
class SequentialList:
def __init__(self, capacity=10):
self.data = [None] * capacity # 初始化存储空间,默认长度为10
self.length = 0 # 线性表当前长度
def insert(self, index, element):
"""在线性表中插入元素"""
if index < 0 or index > self.length:
raise IndexError("Index out of range")
if self.length == len(self.data):
raise OverflowError("Sequential list is full")
for i in range(self.length, index, -1):
self.data[i] = self.data[i-1]
self.data[index] = element
self.length += 1
def delete(self, index):
"""删除线性表中的元素"""
if index < 0 or index >= self.length:
raise IndexError("Index out of range")
element = self.data[index]
for i in range(index, self.length - 1):
self.data[i] = self.data[i+1]
self.data[self.length - 1] = None
self.length -= 1
return element
def get_element(self, index):
"""获取线性表中指定位置的元素"""
if index < 0 or index >= self.length:
raise IndexError("Index out of range")
return self.data[index]
def print_list(self):
"""打印线性表"""
print(self.data[:self.length])
# 使用示例
seq_list = SequentialList()
seq_list.insert(0, 1)
seq_list.insert(1, 2)
seq_list.insert(2, 3)
seq_list.print_list() # 输出: [1, 2, 3]
seq_list.delete(1)
seq_list.print_list() # 输出: [1, 3]
```
在这个例子中,`SequentialList` 类代表了一个顺序存储的线性表,具备插入、删除、获取元素和打印线性表的基本操作。
阅读全文
相关推荐
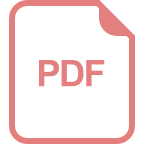
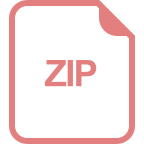


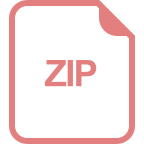
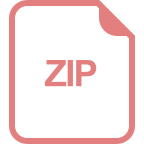
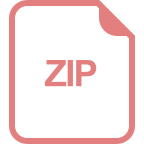
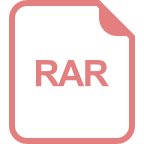
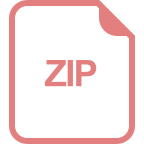
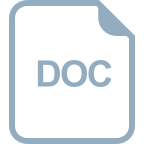
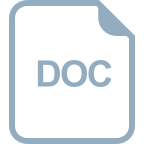


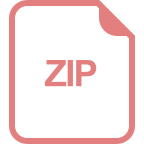