基于verilog的2ASK调制NCU核
时间: 2024-12-25 22:20:16 浏览: 11
### 基于 Verilog 实现 2ASK 调制 NCU 核设计与实现
#### 1. 模块概述
在基于 FPGA 的 2ASK 调制解调系统中,NCU (Numerically Controlled Oscillator) 是用于生成精确频率载波的关键组件。该模块通过数值控制振荡器来产生所需的正弦波形作为载波信号。
#### 2. NCU 模块功能描述
为了实现 2ASK 调制中的载波生成部分,需要构建一个能够根据输入参数调整输出频率的 NCO 单元。此单元接收来自外部控制器设定的目标频率值,并据此计算相位增量 Δφ 来更新内部累加器的状态。每次时钟上升沿到来时,累加器会增加 Δφ 并将其结果送入查表电路(LUT),从而获得对应角度下的正弦函数样本点并最终形成连续变化的模拟量输出[^1]。
```verilog
// NCO Module Implementation in Verilog
module nco (
input wire clk,
input wire rst_n,
input wire [7:0] freq_word, // Frequency control word from external source
output reg signed [9:0] sin_out // Sine wave output with amplitude range [-511,+511]
);
localparam PHASE_WIDTH = 16;
localparam LUT_SIZE = 1 << PHASE_WIDTH;
integer i;
reg [PHASE_WIDTH-1 : 0] phase_acc; // Phase accumulator register
reg [PHASE_WIDTH-1 : 0] next_phase_acc; // Next state of the phase accumulator
// Lookup table initialization for sine values
reg signed [9:0] lut[LUT_SIZE/4 - 1:0];
initial begin
for(i=0;i<LUT_SIZE/4;i=i+1)
lut[i]=10'sd$(sin(2*PI*i/(LUT_SIZE/4))*512); // Pre-compute and store sine samples scaled by factor 512
end
always @(posedge clk or negedge rst_n) begin
if (!rst_n) begin
phase_acc <= {PHASE_WIDTH{1'b0}};
end else begin
phase_acc <= next_phase_acc;
end
end
always @(*) begin
next_phase_acc = phase_acc + {{8{freq_word[7]}}, freq_word}; // Sign extend frequency word before addition
case(phase_acc[PHASE_WIDTH-1 -: 2]) // Select appropriate quadrant based on MSBs of phase acc.
2'b00: sin_out = lut[phase_acc >> 2]; // Quadrant I
2'b01: sin_out = ~lut[(~phase_acc) >> 2]+1; // Quadrant II using symmetry property
2'b10: sin_out = -(lut[(~phase_acc)+1]>>2)-1; // Quadrant III using odd function properties
default:sin_out=~(-lut[-((phase_acc)+1)>>2])-1; // Quadrant IV combining both symmetries
endcase
end
endmodule
```
上述代码展示了如何利用查找表方法高效地近似合成所需频率的正弦波形。这里假设使用了一个具有固定长度(由 `LUT_SIZE` 定义)的一维数组存储预先计算好的离散时间正弦序列片段。每当接收到新的时钟边沿事件时,程序就会依据当前累积相位角索引访问相应的表格项以获取即时采样点数据[^2]。
#### 3. 结合 2ASK 调制逻辑
对于完整的 2ASK 发射机而言,在完成前述 NCO 构建之后还需要加入额外的功能模块负责处理实际的数据流转换过程——即将待传输比特串映射到相应电平状态上并与之前准备好的高频周期性波动相结合。具体来说就是当遇到逻辑 '1' 符号时允许完整强度版本的载波穿过而面对零则切断任何可能存在的干扰成分传递路径。
```verilog
// Combining ASK Modulation Logic With Generated Carrier Waveform From NCO Unit
module two_asks_modulator(
input wire clk,
input wire rst_n,
input wire data_in, // Input binary stream to be modulated
input wire [7:0] freq_word, // Control signal determining carrier frequency via NCO block
output reg out_signal // Final modulated waveform ready for transmission over channel medium
);
wire signed [9:0] carrier_wave; // Intermediate connection carrying generated sinusoidal pattern
nco uut (.clk(clk), .rst_n(rst_n), .freq_word(freq_word), .sin_out(carrier_wave));
always @(posedge clk or negedge rst_n) begin
if (!rst_n) begin
out_signal <= 1'b0;
end else begin
out_signal <= |data_in ? ((carrier_wave >= 0) ^ 1'b1): 1'b0 ; // Perform modulation operation according to incoming bit value
end
end
endmodule
```
这段扩展后的 RTL 描述不仅包含了前面介绍过的 NCO 组件实例化语句而且还定义了一种简单却有效的机制用来执行二进制幅度键控操作。注意这里的表达式采用了三目运算符形式以便更直观清晰地反映出条件判断关系以及后续动作之间的因果联系。
阅读全文
相关推荐
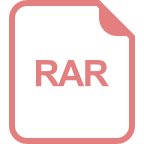




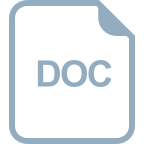

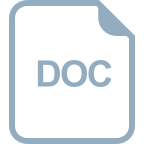


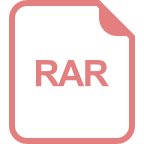







