首页springboot + mybatisplus分页查询
springboot + mybatisplus分页查询
时间: 2023-11-24 14:49:15 浏览: 96
Spring Boot是一个基于Spring框架的快速开发Web应用程序的框架,而Mybatis Plus是Mybatis的增强工具,可以简化Mybatis的开发流程。在Spring Boot中使用Mybatis Plus进行分页查询,需要进行以下步骤:
1. 在pom.xml文件中添加Mybatis Plus和PageHelper的依赖。
2. 编写Mybatis Plus的配置类,其中包括分页插件的配置。
3. 在Mapper接口中编写分页查询的方法。
4. 在Controller中调用Mapper接口中的分页查询方法,并将查询结果返回给前端。
具体实现可以参考引用中提供的代码资源。其中,前端使用Vue和ElementUI进行开发,后端使用Spring Boot和Mybatis Plus进行开发,数据库使用MySQL。在Mybatis Plus的配置类中,使用PaginationInterceptor进行分页插件的配置。在Mapper接口中,使用Mybatis Plus提供的分页查询方法进行分页查询。在Controller中,使用@RequestParam注解获取前端传递的分页参数,并调用Mapper接口中的分页查询方法进行查询。
阅读全文
CSDN会员
开通CSDN年卡参与万元壕礼抽奖
最新推荐
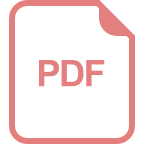
完美解决MybatisPlus插件分页查询不起作用总是查询全部数据问题
问题在于,当你在使用MybatisPlus进行分页查询时,如果未正确配置PaginationInterceptor,分页参数将无法生效,你会观察到所有的数据都被查询出来。要解决这个问题,你需要在你的项目配置中添加...
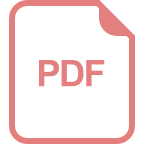
SpringBoot集成MybatisPlus报错的解决方案
SpringBoot集成MybatisPlus报错的解决方案 SpringBoot是一款基于Java的开源框架,旨在简化基于Spring的开发体验,而MybatisPlus是Mybatis的升级版本,提供了许多实用的功能来简化数据库操作。然而,在将MybatisPlus...
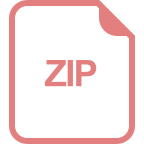
GitHub图片浏览插件:直观展示代码中的图像
资源摘要信息: "ImagesOnGitHub-crx插件"
知识点概述:
1. 插件功能与用途
2. 插件使用环境与限制
3. 插件的工作原理
4. 插件的用户交互设计
5. 插件的图标和版权问题
6. 插件的兼容性
1. 插件功能与用途
插件"ImagesOnGitHub-crx"设计用于增强GitHub这一开源代码托管平台的用户体验。在GitHub上,用户可以浏览众多的代码仓库和项目,但GitHub默认情况下在浏览代码仓库时,并不直接显示图像文件内容,而是提供一个“查看原始文件”的链接。这使得用户体验受到一定限制,特别是对于那些希望直接在网页上预览图像的用户来说不够方便。该插件正是为了解决这一问题,允许用户在浏览GitHub上的图像文件时,无需点击链接即可直接在当前页面查看图像,从而提供更为流畅和直观的浏览体验。
2. 插件使用环境与限制
该插件是专为使用GitHub的用户提供便利的。它能够在GitHub的代码仓库页面上发挥作用,当用户访问的是图像文件页面时。值得注意的是,该插件目前只支持".png"格式的图像文件,对于其他格式如.jpg、.gif等并不支持。用户在使用前需了解这一限制,以免在期望查看其他格式文件时遇到不便。
3. 插件的工作原理
"ImagesOnGitHub-crx"插件的工作原理主要依赖于浏览器的扩展机制。插件安装后,会监控用户在GitHub上的操作。当用户访问到图像文件对应的页面时,插件会通过JavaScript检测页面中的图像文件类型,并判断是否为支持的.png格式。如果是,它会在浏览器地址栏的图标位置上显示一个小octocat图标,用户点击这个图标即可触发插件功能,直接在当前页面上查看到图像。这一功能的实现,使得用户无需离开当前页面即可预览图像内容。
4. 插件的用户交互设计
插件的用户交互设计体现了用户体验的重要性。插件通过在地址栏中增加一个小octocat图标来提示用户当前页面有图像文件可用,这是一种直观的视觉提示。用户通过简单的点击操作即可触发查看图像的功能,流程简单直观,减少了用户的学习成本和操作步骤。
5. 插件的图标和版权问题
由于插件设计者在制作图标方面经验不足,因此暂时借用了GitHub的标志作为插件图标。插件的作者明确表示,如果存在任何错误或版权问题,将会进行更改。这体现了开发者对知识产权尊重的态度,同时也提醒了其他开发者在使用或设计相关图标时应当考虑到版权法律的约束,避免侵犯他人的知识产权。
6. 插件的兼容性
插件的兼容性是评估其可用性的重要标准之一。由于插件是为Chrome浏览器的用户所设计,因此它使用了Chrome扩展程序的标准格式,即.crx文件。用户需要通过浏览器的扩展程序管理界面进行安装。尽管目前插件仅支持.png图像格式,但对于希望在GitHub上浏览.png图像文件的用户来说,已经提供了非常实用的功能。未来,若开发者计划拓展插件支持的文件格式或适用于其他浏览器,则需要考虑到对现有代码的扩展和兼容性测试。
总结:
"ImagesOnGitHub-crx"插件通过创新的用户体验设计,解决了GitHub在浏览图像文件时的一些局限性,使得图像浏览更加直观和便捷。尽管目前该插件存在一些限制,如仅支持.png格式和仅在Chrome浏览器中可用,但它为用户和开发者提供了良好的思路和实践。对于希望提高效率和增强功能的用户来说,这类工具扩展了GitHub的实用性,是开发人员工具箱中的一个有益补充。

管理建模和仿真的文件
管理Boualem Benatallah引用此版本:布阿利姆·贝纳塔拉。管理建模和仿真。约瑟夫-傅立叶大学-格勒诺布尔第一大学,1996年。法语。NNT:电话:00345357HAL ID:电话:00345357https://theses.hal.science/tel-003453572008年12月9日提交HAL是一个多学科的开放存取档案馆,用于存放和传播科学研究论文,无论它们是否被公开。论文可以来自法国或国外的教学和研究机构,也可以来自公共或私人研究中心。L’archive ouverte pluridisciplinaire
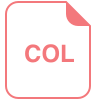
【OPPO手机故障诊断专家】:工程指令快速定位与解决
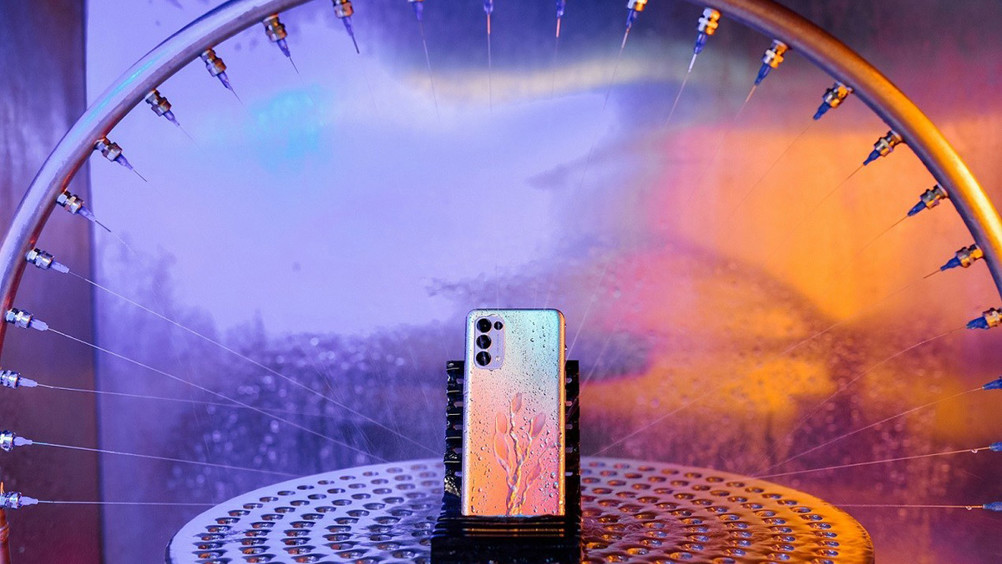
# 摘要
本文综述了OPPO手机故障诊断的技术细节,涵盖了工程指令的基础理论、实践应用、高级技巧以及未来发展方向。首先介绍了工程指令的定义、分类、执行环境及其与手机系统交互的重要性。随后,深入探讨了工程指令在初步故障诊断

求[100,900]之间相差为12的素数对(注:要求素数对的两个素数均在该范围内)的个数
求解 [100, 900] 范围内相差为 12 的素数对,首先我们需要确定哪些数在这个区间内是素数。然后筛选出它们成对出现且差值为 12 的情况。
1. 确定素数范围内的素数:我们可以编写一个简单的程序来检查每个数字是否为素数,如果数字大于 1,并且除 2 到其平方根之间的所有整数都不能整除它,那么这个数字就是素数。
2. 遍历并寻找符合条件的素数对:从较大的素数开始向下遍历,找到的第一个素数作为“较大”素数,然后查看比它小 12 的下一个数,如果这个数也是素数,则找到了一对符合条件的素数。
3. 统计素数对的数量:统计在给定范围内找到的这种差距为 12 的素数对的数量。
由于计算素数
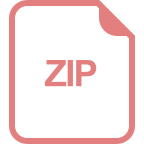
Android IPTV项目:直播频道的实时流媒体实现
资源摘要信息:"IPTV:直播IPTV的Android项目是一个基于Android平台的实时流式传输应用。该项目允许用户从M3U8或M3U格式的链接或文件中获取频道信息,并将这些频道以网格或列表的形式展示。用户可以在应用内选择并播放指定的频道。该项目的频道列表是从一个预设的列表中加载的,并且通过解析M3U或M3U8格式的文件来显示频道信息。开发者还计划未来更新中加入Exo播放器以及电子节目单功能,以增强用户体验。此项目使用了多种技术栈,包括Java、Kotlin以及Kotlin Android扩展。"
知识点详细说明:
1. IPTV技术:
IPTV(Internet Protocol Television)即通过互联网协议提供的电视服务。它与传统的模拟或数字电视信号传输方式不同,IPTV通过互联网将电视内容以数据包的形式发送给用户。这种服务使得用户可以按需观看电视节目,包括直播频道、视频点播(VOD)、时移电视(Time-shifted TV)等。
2. Android开发:
该项目是针对Android平台的应用程序开发,涉及到使用Android SDK(软件开发工具包)进行应用设计和功能实现。Android应用开发通常使用Java或Kotlin语言,而本项目还特别使用了Kotlin Android扩展(Kotlin-Android)来优化开发流程。
3. 实时流式传输:
实时流式传输是指媒体内容以连续的流形式进行传输的技术。在IPTV应用中,实时流式传输保证了用户能够及时获得频道内容。该项目可能使用了HTTP、RTSP或其他流媒体协议来实现视频流的实时传输。
4. M3U/M3U8文件格式:
M3U(Moving Picture Experts Group Audio Layer 3 Uniform Resource Locator)是一种常用于保存播放列表的文件格式。M3U8则是M3U格式的扩展版本,支持UTF-8编码,常用于苹果设备。在本项目中,M3U/M3U8文件被用来存储IPTV频道信息,如频道名称、视频流URL等。
5. Exo播放器:
ExoPlayer是谷歌官方提供的一个开源视频播放器,专为Android优化。它支持多种特性,如自定义字幕、HDR视频播放、无缝直播等。ExoPlayer通常用于处理IPTV应用中的视频流媒体播放需求。
6. 电子节目单(EPG):
电子节目单是IPTV应用中一项重要功能,它为用户提供频道的节目指南,包括当前播放的节目以及未来节目的安排。电子节目单一般以网格或列表形式展示,方便用户浏览和搜索节目信息。
7. 开源贡献文化:
该项目提到了欢迎贡献者,表明这是一个开源项目。在开源文化中,开发者社区鼓励用户、开发者贡献代码来改进项目,这是一个共享知识、共同进步的过程。参与者通过贡献代码、报告问题或提供文档帮助等方式参与项目。
8. Kotlin编程语言:
Kotlin是一种运行在Java虚拟机上的静态类型编程语言,它与Java完全兼容并可以无缝集成Java代码。Kotlin以其简洁、安全和富有表现力的特点被越来越多的Android开发者采用。在本项目中,使用Kotlin可以简化代码结构,提高开发效率和应用性能。
总结而言,本项目是一个面向Android平台的实时流媒体IPTV应用开发项目,它整合了实时流式传输、M3U/M3U8文件解析、Exo播放器使用、电子节目单功能等关键技术点,并在开源社区中寻求贡献者的参与。通过本项目,开发者可以深入了解如何在Android平台上实现IPTV服务,并学习到使用Kotlin和Java等编程语言进行Android应用开发的相关知识。

"互动学习:行动中的多样性与论文攻读经历"
多样性她- 事实上SCI NCES你的时间表ECOLEDO C Tora SC和NCESPOUR l’Ingén学习互动,互动学习以行动为中心的强化学习学会互动,互动学习,以行动为中心的强化学习计算机科学博士论文于2021年9月28日在Villeneuve d'Asq公开支持马修·瑟林评审团主席法布里斯·勒菲弗尔阿维尼翁大学教授论文指导奥利维尔·皮耶昆谷歌研究教授:智囊团论文联合主任菲利普·普雷教授,大学。里尔/CRISTAL/因里亚报告员奥利维耶·西格德索邦大学报告员卢多维奇·德诺耶教授,Facebook /索邦大学审查员越南圣迈IMT Atlantic高级讲师邀请弗洛里安·斯特鲁布博士,Deepmind对于那些及时看到自己错误的人...3谢谢你首先,我要感谢我的两位博士生导师Olivier和Philippe。奥利维尔,"站在巨人的肩膀上"这句话对你来说完全有意义了。从科学上讲,你知道在这篇论文的(许多)错误中,你是我可以依
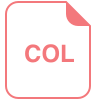
【OPPO手机工程模式终极指南】:掌握这些秘籍,故障排查不再难!
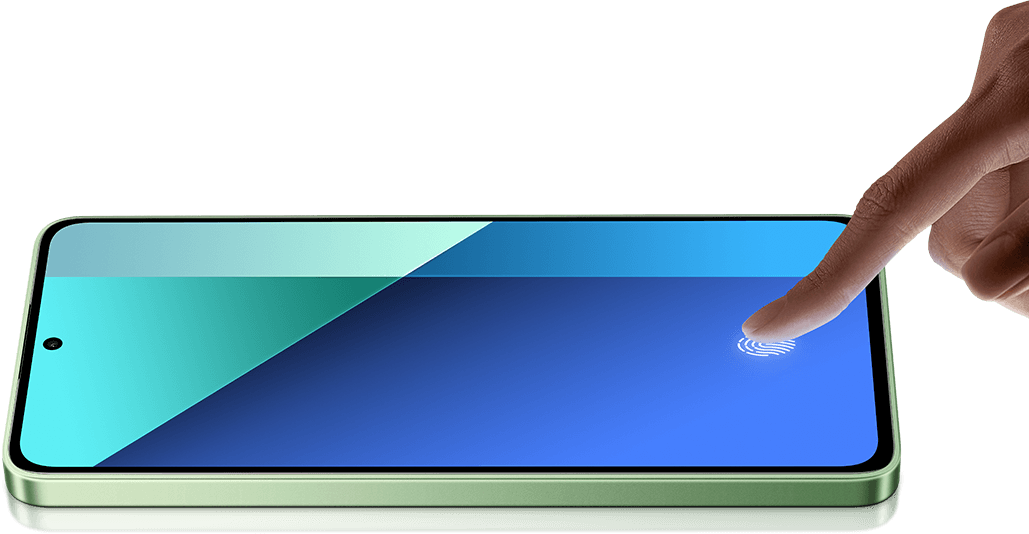
# 摘要
OPPO手机工程模式是为高级用户和开发者设计的一组调试和诊断工具集,它能够帮助用户深入了解手机硬件信息、进行测试和故障诊断,并优化设备性能。本文将对OPPO工程模式进行系统性的介绍,包括如何进入和安全退出该模式,详述其中的基础与高级功能,并提供实用的故障诊断和排查技巧。同时,本文还将探讨如何利用工程模式对

前端在json文件里写模板,可以换行 有空格现在在文本框的时候
前端在 JSON 文件中通常不会直接写模板,并且 JSON 格式本身是基于键值对的,不支持换行和空格的保留。JSON 是一种数据交换格式,它要求数据结构紧凑、易于解析。如果你需要在前端显示模板内容,推荐使用 JavaScript 的模板字符串 (Template literals) 或者 Handlebars、Mustache 等前端模板引擎。
当你想在文本框中显示 JSON 数据时,会先将 JSON 转换成 HTML 可渲染的内容。例如:
```javascript
let jsonData = {
"template": "这是一个<br>换行示例",
"text": "这是文