vue3+ts写一个上传图片到数据库
时间: 2024-01-14 10:02:16 浏览: 27
首先,需要在后端编写接口来处理图片上传,并返回图片的访问地址。这里以 Node.js + Express + Multer 为例。
1. 安装相关依赖
```
npm install express multer
```
2. 编写接口
```typescript
import express from 'express';
import multer from 'multer';
const app = express();
const upload = multer({ dest: 'uploads/' }); // 上传文件存储目录
app.post('/upload', upload.single('file'), (req, res) => {
const { filename } = req.file;
const url = `http://localhost:3000/uploads/${filename}`; // 访问图片的地址
// 将图片的访问地址存入数据库
res.send({ url });
});
app.listen(3000, () => {
console.log('Server is running at http://localhost:3000');
});
```
3. 在前端中使用 axios 发送请求
```typescript
import { ref } from 'vue';
import axios from 'axios';
export default {
setup() {
const fileRef = ref(null);
const imageUrlRef = ref('');
const uploadImage = async () => {
const formData = new FormData();
formData.append('file', fileRef.value);
try {
const response = await axios.post('/upload', formData, {
headers: {
'Content-Type': 'multipart/form-data',
},
});
imageUrlRef.value = response.data.url;
} catch (error) {
console.error(error);
}
};
return {
fileRef,
imageUrlRef,
uploadImage,
};
},
};
```
4. 使用组件上传图片
```html
<template>
<div>
<input type="file" ref="fileRef" @change="uploadImage">
<img :src="imageUrlRef">
</div>
</template>
<script>
import { ref } from 'vue';
import axios from 'axios';
export default {
setup() {
const fileRef = ref(null);
const imageUrlRef = ref('');
const uploadImage = async () => {
const formData = new FormData();
formData.append('file', fileRef.value);
try {
const response = await axios.post('/upload', formData, {
headers: {
'Content-Type': 'multipart/form-data',
},
});
imageUrlRef.value = response.data.url;
} catch (error) {
console.error(error);
}
};
return {
fileRef,
imageUrlRef,
uploadImage,
};
},
};
</script>
```
相关推荐
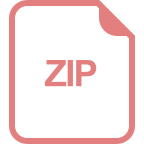
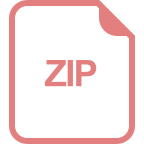
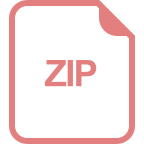














