pygame添加图标按键
时间: 2024-11-22 22:35:07 浏览: 17
在Pygame库中,添加图标按键主要是为了提升用户界面的可视化效果,使其更具吸引力。你可以通过以下几个步骤来完成:
1. **导入所需模块**:
首先,确保已经导入了`pygame`和`pygame.image`模块,因为它们包含了图像处理功能。
```python
import pygame
from pygame.locals import *
```
2. **加载图标图片**:
使用`pygame.image.load()`函数加载你的图标图片文件,例如 `.png` 或 `.jpg` 等格式。
```python
icon = pygame.image.load('path_to_your_icon.png')
```
3. **创建按钮类**:
定义一个包含图标和文本的按钮类,通常有位置、尺寸、点击事件处理等属性和方法。
```python
class IconButton(pygame.sprite.Sprite):
def __init__(self, x, y, icon, text):
super().__init__()
self.image = icon # 图标
self.text_image = pygame.font.SysFont(None, 30).render(text, True, (0, 0, 0))
self.rect = self.image.get_rect() # 获取图标矩形
self.rect.center = (x, y) # 设置中心点位置
def draw(self, window):
window.blit(self.image, self.rect)
window.blit(self.text_image, (self.rect.x + 5, self.rect.y + 5)) # 文本位置调整
def update(self, pos):
if self.rect.collidepoint(pos): # 检查是否点击到按钮区域
# 这里可以添加点击事件处理代码
print("Button clicked!")
```
4. **在游戏循环中使用**:
在主的游戏循环中,实例化这个按钮,并在适当的位置绘制和更新它。
```python
# 创建并添加按钮
button = IconButton(50, 50, icon, "Click Me")
all_sprites.add(button)
# 游戏主循环
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.MOUSEBUTTONDOWN:
button.update(event.pos)
all_sprites.draw(screen)
screen.fill((255, 255, 255)) # 清屏并绘制背景
pygame.display.flip()
```
阅读全文
相关推荐
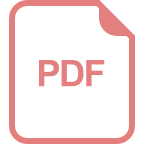
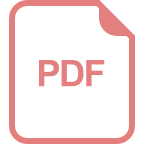
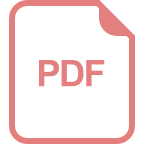

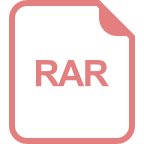
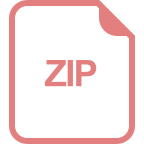
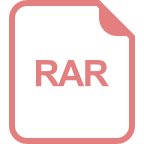
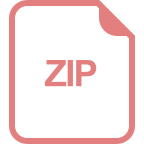
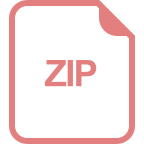
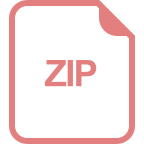
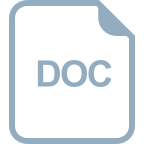
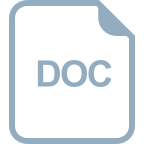
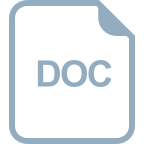
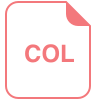
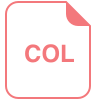


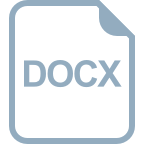
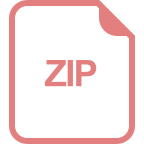