在vue中使用jsPDF组件将多个pdf合并为一个
时间: 2023-12-14 15:37:48 浏览: 76
首先,你需要安装jsPDF组件。可以使用npm命令进行安装:
```
npm install jspdf --save
```
接下来,你可以使用jsPDF的addPDF方法将多个PDF文件合并为一个。这个方法需要将PDF文件的url作为参数传递给它。所以,你需要先加载所有的PDF文件并将它们的url存储在一个数组中。
下面是一个简单的示例代码,演示如何使用jsPDF将多个PDF文件合并为一个:
```javascript
import jsPDF from 'jspdf';
const urls = ['url1.pdf', 'url2.pdf', 'url3.pdf'];
// create a new instance of jsPDF
const doc = new jsPDF();
// loop through the array of PDF urls
urls.forEach((url, index) => {
// fetch the PDF file from the url
fetch(url)
.then(response => response.blob())
.then(blob => {
// convert the blob into a data URL
const dataUrl = URL.createObjectURL(blob);
// add the PDF to the jsPDF document
doc.addPage();
doc.addPDF(dataUrl, 'all');
// if this is the last PDF, save and download the merged document
if (index === urls.length - 1) {
doc.save('merged.pdf');
}
});
});
```
上面的代码做了以下几个事情:
1. 导入jsPDF组件。
2. 创建一个包含所有PDF文件url的数组。
3. 创建一个新的jsPDF实例。
4. 循环遍历PDF文件url数组。
5. 从url中获取PDF文件。
6. 将获取到的PDF文件转换成data URL。
7. 将转换后的data URL添加到jsPDF文档中。
8. 如果这是最后一个PDF文件,则保存和下载合并后的文档。
请注意,上面的示例代码使用了ES6的箭头函数和模板字符串。如果你的项目不支持这些语言特性,你需要相应地修改代码。
相关推荐
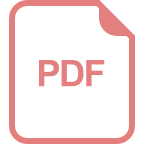
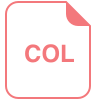
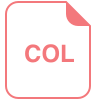












