java中String怎么遍历
时间: 2024-06-11 18:04:06 浏览: 3
可以通过以下两种方式遍历一个String对象:
1. 使用charAt()方法
使用charAt()方法可以获取字符串中每个字符的Unicode值。
示例代码:
```
String str = "Hello, world!";
for (int i = 0; i < str.length(); i++) {
char c = str.charAt(i);
System.out.println(c);
}
```
2. 将字符串转换为字符数组
可以使用toCharArray()方法将字符串转换为字符数组,然后遍历该字符数组。
示例代码:
```
String str = "Hello, world!";
char[] charArray = str.toCharArray();
for (char c : charArray) {
System.out.println(c);
}
```
相关问题
java 获取string 遍历8位数字
如果你想从一个字符串中获取8位数字并进行遍历,可以使用正则表达式的方式来实现。具体代码如下:
```
String str = "这是一个123456789的字符串,里面还有98765432";
Pattern pattern = Pattern.compile("\\d{8}");
Matcher matcher = pattern.matcher(str);
while (matcher.find()) {
String matchStr = matcher.group();
for (int i = 0; i < matchStr.length(); i++) {
char ch = matchStr.charAt(i);
System.out.println(ch);
}
}
```
这里的正则表达式 "\\d{8}" 表示匹配8个数字,然后用 Matcher 对象对字符串进行匹配。如果匹配成功,就获取匹配到的字符串,然后对其进行遍历输出。
Java中map遍历
在Java中,我们有多种方式可以遍历Map。根据提供的引用内容,我们可以使用forEach方法或者使用entrySet方法结合for循环来遍历Map。
使用forEach方法遍历Map的代码如下:
```
Map<String, Integer> maps = new HashMap<>();
maps.put("华为", 10);
maps.put("小米", 5);
maps.put("iPhone", 6);
maps.put("生活用品", 15);
maps.put("java", 20);
maps.put("python", 17);
maps.forEach((key, value) -> System.out.println(key + " ---> " + value));
```
使用entrySet方法结合for循环遍历Map的代码如下:
```
Map<String, Integer> maps = new HashMap<>();
maps.put("华为", 10);
maps.put("小米", 5);
maps.put("iPhone", 6);
maps.put("生活用品", 15);
maps.put("java", 20);
maps.put("python", 17);
Set<Map.Entry<String, Integer>> entrySet = maps.entrySet();
for (Map.Entry<String, Integer> entry : entrySet) {
String key = entry.getKey();
Integer value = entry.getValue();
System.out.println(key + " ---> " + value);
}
```
以上是两种常用的遍历Map的方法,根据你的需求选择适合的方法即可。
相关推荐
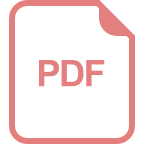
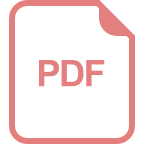
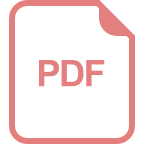












