vue3 ts 通过xlsx实现网页预览 base64 excel 文件 并显示原excel样式
时间: 2024-03-21 08:38:19 浏览: 16
您好!对于您的问题,我可以提供以下思路:
1. 使用 xlsx 库解析 base64 编码的 excel 文件,将其转换为 JSON 数据;
2. 使用 vue3 的 template 或 JSX 语法,结合样式库,渲染出表格的 HTML 结构;
3. 针对 excel 文件中的样式,使用 CSS 样式或 JavaScript 动态添加样式,实现表格的样式效果。
具体实现细节,可以参考以下代码示例:
```
<template>
<div class="table-container">
<table class="table">
<thead>
<tr>
<th v-for="(col, index) in columns" :key="index">{{ col }}</th>
</tr>
</thead>
<tbody>
<tr v-for="(row, index) in rows" :key="index">
<td v-for="(cell, index) in row" :key="index">{{ cell }}</td>
</tr>
</tbody>
</table>
</div>
</template>
<script lang="ts">
import { defineComponent } from 'vue';
import * as XLSX from 'xlsx';
export default defineComponent({
props: {
fileData: {
type: String,
required: true,
},
},
data() {
return {
columns: [], // 表格列名
rows: [], // 表格数据
};
},
mounted() {
// 解析 base64 编码的 excel 文件
const file = XLSX.read(this.fileData, { type: 'base64' });
const sheet = file.Sheets[file.SheetNames[0]];
const data = XLSX.utils.sheet_to_json(sheet, { header: 1 });
// 提取表格列名和数据
this.columns = data[0];
this.rows = data.slice(1);
},
});
</script>
<style scoped>
.table-container {
overflow-x: auto;
}
.table {
border-collapse: collapse;
width: 100%;
}
.table th,
.table td {
border: 1px solid #ddd;
padding: 8px;
text-align: left;
}
.table th {
background-color: #f2f2f2;
}
</style>
```
这个组件可以接收一个 base64 编码的 excel 文件,解析出其中的表格数据和样式,并渲染出一个带有原样式的表格。您只需要将该组件引入到您的项目中,并传递正确的参数,即可实现您想要的预览效果。
希望我的回答能对您有所帮助!如果您还有其他问题,欢迎继续提问。
相关推荐
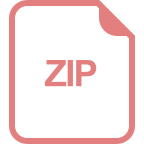
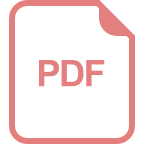
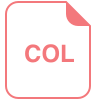
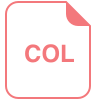
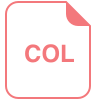
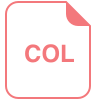










