c++ try catch 如何获得异常的所在行号
时间: 2023-06-21 18:22:24 浏览: 830
在 C++ 中,我们可以通过在 catch 块中捕获异常并使用标准库中的 std::exception 类型来获取异常信息。然而,要获取异常发生的行号,需要在抛出异常前使用 __LINE__ 预处理器宏将当前行号嵌入到异常中。具体实现可以参考以下代码:
```c++
try {
// some code that may throw an exception
} catch(std::exception& e) {
std::cout << "Exception caught: " << e.what() << " at line " << __LINE__ << std::endl;
}
```
在这个例子中,我们在 catch 块中打印出异常信息和发生异常的行号。注意,__LINE__ 是一个预处理器宏,它会在编译时被替换为当前的行号。因此,我们需要在抛出异常前使用它来将行号嵌入到异常信息中。
相关问题
c++ try catch 如何获得异常的所在具体错误行号
在 C++ 中,可以使用标准库中的 `std::exception` 异常类和 `try-catch` 语句来捕获并处理异常。如果想要在异常处理中获取异常发生的具体错误行号,可以使用 `std::exception` 类的 `what()` 函数来获取异常的错误信息。
要想在错误信息中包含具体错误行号,可以在抛出异常时使用 `__LINE__` 预处理宏来获取当前代码行号,并将其作为错误信息的一部分抛出。例如:
```
try {
// some code that may throw an exception
} catch (const std::exception& e) {
std::cerr << "Error at line " << __LINE__ << ": " << e.what() << std::endl;
}
```
这样,在捕获异常时,就可以获取到发生异常的具体错误行号,并将其与异常信息一起输出。
c++怎么在try{}catch(...){}中得到代码停止执行的位置(行号)?
在C++中,可以使用`__LINE__`和`__FILE__`宏来获取代码行号和文件名。在`catch(...)`块中,你可以使用`std::exception_ptr`来保存当前发生的异常,然后在捕获到异常时使用`std::current_exception()`来获取异常指针。然后通过`std::exception_ptr`的`what()`方法获取异常信息,并使用`__LINE__`和`__FILE__`宏来获取当前的代码行号和文件名。
以下是一个示例代码:
```c++
#include <iostream>
#include <exception>
#include <string>
int main() {
try {
int a = 10;
int b = 0;
int c = a / b;
}
catch (...) {
std::exception_ptr p = std::current_exception();
try {
if (p) {
std::rethrow_exception(p);
}
}
catch (const std::exception& e) {
std::cerr << "Exception caught at " << __FILE__ << ":" << __LINE__ << std::endl;
std::cerr << e.what() << std::endl;
}
}
return 0;
}
```
在上面的示例中,由于除数为0,会抛出一个`std::exception`异常,并在`catch(...)`块中捕获。然后使用`std::current_exception()`获取异常指针,并在内部`try-catch`块中调用`std::rethrow_exception()`重新引发异常。最后,在`catch(const std::exception& e)`块中获取异常信息并输出行号和文件名。
阅读全文
相关推荐
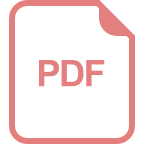
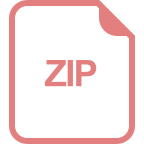
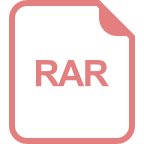
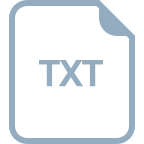
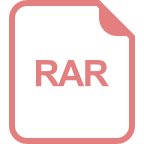
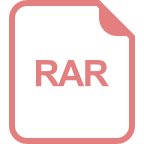
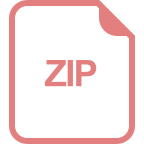
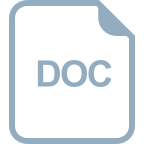
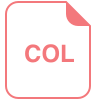
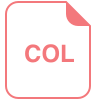
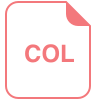
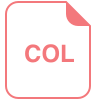
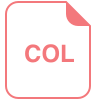
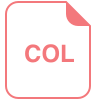
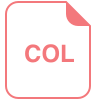

