java给出两个整数集合A、B,求出他们的交集、并集以及B在A中的余集。 输入 第一行为一个整数n,表示集合A中的元素个数。 第二行有n个互不相同的用空格隔开的整数,表示集合A中的元素。 第三行为一个整数m,表示集合B中的元素个数。 第四行有m个互不相同的用空格隔开的整数,表示集合B中的元素。 集合中的所有元素均为int范围内的整数,n、m<=1000。 输出 第一行按从小到大的顺序输出A、B交集中的所有元素。 第二行按从小到大的顺序输出A、B并集中的所有元素。 第三行按从小到大的顺序输出B在A中的余集中的所有元素
时间: 2024-03-03 20:49:54 浏览: 122
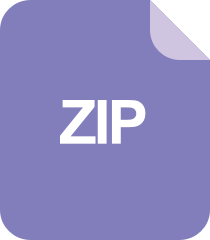
用于处理数据,求两个集合的交集、并集、补集。附源码。
好的,这是一道关于集合操作的题目,我可以为您提供Java代码解决这个问题。请确认您已经将题目中的输入数据输入到程序中的变量中,然后您可以使用以下代码来解决这个问题:
```java
import java.util.*;
public class SetOperations {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
Set<Integer> setA = new HashSet<>();
for (int i = 0; i < n; i++) {
setA.add(sc.nextInt());
}
int m = sc.nextInt();
Set<Integer> setB = new HashSet<>();
for (int i = 0; i < m; i++) {
setB.add(sc.nextInt());
}
// 交集
Set<Integer> intersection = new HashSet<>(setA);
intersection.retainAll(setB);
List<Integer> intersectionList = new ArrayList<>(intersection);
Collections.sort(intersectionList);
for (int i : intersectionList) {
System.out.print(i + " ");
}
System.out.println();
// 并集
Set<Integer> union = new HashSet<>(setA);
union.addAll(setB);
List<Integer> unionList = new ArrayList<>(union);
Collections.sort(unionList);
for (int i : unionList) {
System.out.print(i + " ");
}
System.out.println();
// B在A中的余集
Set<Integer> complement = new HashSet<>(setA);
complement.removeAll(setB);
List<Integer> complementList = new ArrayList<>(complement);
Collections.sort(complementList);
for (int i : complementList) {
System.out.print(i + " ");
}
System.out.println();
}
}
```
这个程序会首先读取两个集合A和B,并使用Java中的Set集合来表示它们。
然后,程序会计算出A和B的交集、并集以及B在A中的余集,并分别将结果存储在intersection、union和complement这三个Set集合中。
最后,程序将这些Set集合转换为List并按从小到大的顺序输出其中的元素。
阅读全文
相关推荐





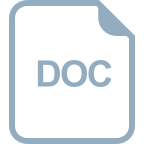









