请帮我写一个mvvm架构的Android应用程序。该程序的功能是:读取一个文本文件计算其中的中文个数,英文单词个数,除去空格以外所有字符个数,包含空格在内所有字符的个数。请实现这个程序的所有函数和功能,完善这个程序
时间: 2024-02-25 18:52:50 浏览: 62
好的,我会帮助您完成这个任务。首先,我们需要了解 MVVM 架构的基本概念和应用。MVVM 是 Model-View-ViewModel 的缩写,它是一种用于构建用户界面的软件架构模式。在 MVVM 中,界面被分为三个部分:
1. Model:模型层,它负责处理数据的存储和操作。
2. View:视图层,它是用户界面的展示部分。
3. ViewModel:视图模型层,它连接视图和模型,负责处理视图和模型之间的通信和逻辑处理。
下面是实现这个应用程序的步骤:
1. 创建一个 Android 项目并设置好基本的界面布局。
2. 创建一个用于存储文件内容的 Model 类,并添加处理数据的函数。
3. 创建一个 ViewModel 类,它将处理视图和模型之间的通信和逻辑处理。在 ViewModel 中,我们需要实现以下功能:
- 读取文本文件并将内容传递给 Model 类进行处理。
- 将 Model 类处理后的数据传递给视图层进行展示。
4. 创建一个 View 类,它将展示数据和用户界面。在 View 类中,我们需要实现以下功能:
- 接收 ViewModel 的数据并将其展示在用户界面上。
- 添加用户界面的事件响应函数,如按钮点击事件等。
下面是一个示例代码,您可以参考它进行实现:
Model 类:
```java
public class FileContentModel {
public static int countChinese(String content) {
int count = 0;
Pattern pattern = Pattern.compile("[\u4e00-\u9fa5]");
Matcher matcher = pattern.matcher(content);
while (matcher.find()) {
count++;
}
return count;
}
public static int countEnglish(String content) {
int count = 0;
Pattern pattern = Pattern.compile("[a-zA-Z]+");
Matcher matcher = pattern.matcher(content);
while (matcher.find()) {
count++;
}
return count;
}
public static int countCharactersWithoutSpaces(String content) {
content = content.replaceAll("\\s+", "");
return content.length();
}
public static int countAllCharacters(String content) {
return content.length();
}
}
```
ViewModel 类:
```java
public class MainViewModel extends ViewModel {
private MutableLiveData<String> mContent = new MutableLiveData<>();
public void readTextFile(String filePath) {
try {
File file = new File(filePath);
FileInputStream fis = new FileInputStream(file);
InputStreamReader isr = new InputStreamReader(fis);
BufferedReader br = new BufferedReader(isr);
StringBuilder sb = new StringBuilder();
String line;
while ((line = br.readLine()) != null) {
sb.append(line);
}
mContent.setValue(sb.toString());
br.close();
} catch (IOException e) {
e.printStackTrace();
}
}
public LiveData<String> getContent() {
return mContent;
}
public LiveData<String> getStatistics() {
MutableLiveData<String> statistics = new MutableLiveData<>();
String content = mContent.getValue();
if (content != null) {
int chineseCount = FileContentModel.countChinese(content);
int englishCount = FileContentModel.countEnglish(content);
int charactersWithoutSpacesCount = FileContentModel.countCharactersWithoutSpaces(content);
int allCharactersCount = FileContentModel.countAllCharacters(content);
statistics.setValue("中文个数:" + chineseCount + "\n英文单词个数:" + englishCount +
"\n除去空格以外所有字符个数:" + charactersWithoutSpacesCount + "\n包含空格在内所有字符的个数:" + allCharactersCount);
}
return statistics;
}
}
```
View 类:
```java
public class MainActivity extends AppCompatActivity {
private MainViewModel mViewModel;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mViewModel = new ViewModelProvider(this).get(MainViewModel.class);
final TextView contentTextView = findViewById(R.id.contentTextView);
final TextView statisticsTextView = findViewById(R.id.statisticsTextView);
final EditText filePathEditText = findViewById(R.id.filePathEditText);
final Button readButton = findViewById(R.id.readButton);
mViewModel.getContent().observe(this, new Observer<String>() {
@Override
public void onChanged(String s) {
contentTextView.setText(s);
}
});
mViewModel.getStatistics().observe(this, new Observer<String>() {
@Override
public void onChanged(String s) {
statisticsTextView.setText(s);
}
});
readButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String filePath = filePathEditText.getText().toString();
mViewModel.readTextFile(filePath);
}
});
}
}
```
以上就是一个基于 MVVM 架构的 Android 应用程序的示例代码,它可以读取一个文本文件并计算其中的中文个数、英文单词个数、除去空格以外所有字符个数、包含空格在内所有字符的个数。您可以根据需要进行修改和完善。
阅读全文
相关推荐
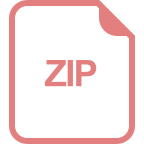
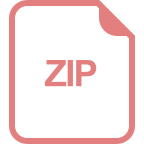
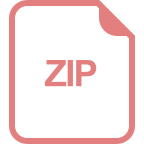
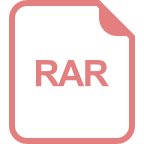
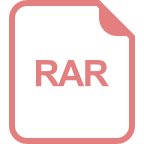
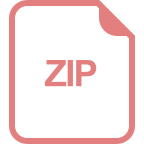
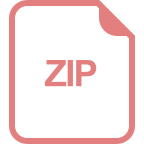
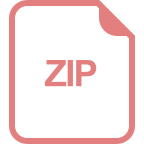
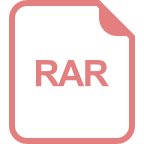
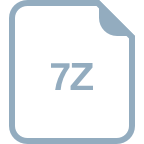
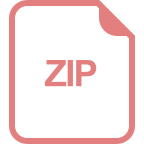
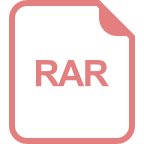
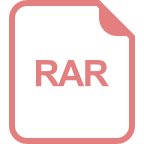
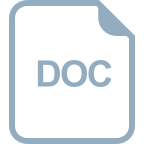
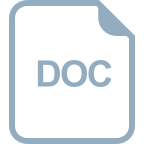
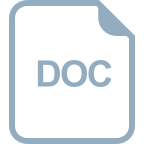