ffmpeg里把RGB转成YUV的Y的权重也是Y = 0.299R + 0.587G + 0.114B吗?
时间: 2023-07-14 17:14:14 浏览: 74
是的,FFmpeg中将RGB转换为YUV时,使用的Y的权重是Y = 0.299R + 0.587G + 0.114B,这个公式也是ITU-R BT.601标准中定义的。但是需要注意的是,不同的标准可能会有不同的YUV转换公式,例如ITU-R BT.709标准使用的Y的权重是Y = 0.2126R + 0.7152G + 0.0722B。因此,在使用FFmpeg进行RGB到YUV转换时,需要根据具体的应用场景选择合适的标准和转换公式。
相关问题
ffmpeg的怎么把RGB转到YUV420的
你可以使用ffmpeg命令行工具将RGB图像转换为YUV420格式。下面是一个示例命令:
```
ffmpeg -i input.rgb -pix_fmt yuv420p output.yuv
```
其中,`input.rgb` 是输入的RGB图像文件,`output.yuv` 是输出的YUV420格式图像文件。`-pix_fmt yuv420p` 参数指定了输出图像的像素格式为YUV420。
如果你需要将多个RGB图像一次性转换为YUV420格式,可以使用类似以下命令:
```
ffmpeg -f rawvideo -pix_fmt rgb24 -s 640x480 -i input_%03d.rgb -pix_fmt yuv420p output.yuv
```
其中,`input_%03d.rgb` 表示多个RGB图像文件名称的通配符,`-s 640x480` 参数指定了RGB图像的分辨率是640x480。
让这个代码一直执行ffmpeg -f x11grab -r 1 -s 800x600 -i :0 -vframes 1 -y output.jpg
很抱歉,我无法执行实际命令,因为我只是一个文本聊天机器人。但是,你可以在终端中执行该命令。该命令将捕获屏幕的一个帧并将其保存为JPEG图像。如果你想一直执行此命令,可以将其包装在一个无限循环中,如下所示:
```
while true; do ffmpeg -f x11grab -r 1 -s 800x600 -i :0 -vframes 1 -y output.jpg; done
```
此命令将不断运行,每秒捕获一次屏幕并将其保存为output.jpg。要停止此命令,请按Ctrl + C。请注意,此命令可能会占用大量系统资源。
相关推荐
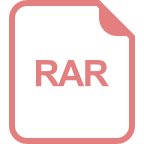
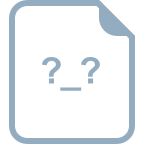
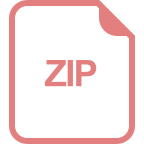












