游戏开发:使用C语言开发一个简单的游戏,如俄罗斯方块、贪吃蛇等。
时间: 2024-02-05 12:11:58 浏览: 119
好的,我可以为您介绍一下使用C语言开发俄罗斯方块的基本步骤。
首先,我们需要了解一下俄罗斯方块游戏的基本规则和玩法。俄罗斯方块游戏中,玩家需要控制一个下落的方块,并将它放置在底部的方块堆中。每个方块由四个小方块组成,玩家可以左右移动方块,也可以旋转方块,直到将它放置在最佳位置。当一行方块被填满时,这一行就会被消除,玩家可以得到相应的分数。如果方块堆的高度超过了屏幕的顶部,游戏就结束了。
接下来,我们需要使用C语言编写游戏代码。我们可以使用C语言中的图形库来实现游戏画面的显示和操作,例如Windows API或者SDL等。同时,我们还需要编写游戏逻辑的代码,包括方块的下落、移动和旋转,以及方块堆的状态判断和更新。最后,我们还需要实现游戏的分数计算和显示,以及游戏结束时的处理。
以下是一个简单的俄罗斯方块游戏的C语言代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <conio.h>
#include <windows.h>
#define MAX_WIDTH 10
#define MAX_HEIGHT 20
int board[MAX_HEIGHT][MAX_WIDTH] = {0};
int score = 0;
int tetromino[7][4][4] = {
{{0,0,0,0},
{0,0,0,0},
{0,0,0,0},
{0,0,0,0}},
{{0,0,0,0},
{1,1,1,1},
{0,0,0,0},
{0,0,0,0}},
{{0,0,0,0},
{0,2,2,0},
{0,2,2,0},
{0,0,0,0}},
{{0,0,0,0},
{0,3,3,0},
{3,3,0,0},
{0,0,0,0}},
{{0,0,0,0},
{4,4,0,0},
{0,4,4,0},
{0,0,0,0}},
{{0,0,0,0},
{0,5,0,0},
{5,5,5,0},
{0,0,0,0}},
{{0,0,0,0},
{0,6,0,0},
{0,6,6,0},
{0,0,6,0}}
};
int current_tetromino[4][4] = {0};
int current_x = 0;
int current_y = 0;
void new_tetromino() {
int type = rand() % 7;
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
current_tetromino[i][j] = tetromino[type][i][j];
}
}
current_x = MAX_WIDTH / 2 - 2;
current_y = 0;
}
void draw_board() {
system("cls");
for (int i = 0; i < MAX_HEIGHT; i++) {
for (int j = 0; j < MAX_WIDTH; j++) {
if (board[i][j] == 0) {
printf(".");
} else {
printf("*");
}
}
printf("\n");
}
printf("Score: %d\n", score);
}
int check_collision(int x, int y) {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (current_tetromino[i][j] != 0) {
int new_x = x + j;
int new_y = y + i;
if (new_x < 0 || new_x >= MAX_WIDTH || new_y >= MAX_HEIGHT) {
return 1;
}
if (new_y >= 0 && board[new_y][new_x] != 0) {
return 1;
}
}
}
}
return 0;
}
void update_board() {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (current_tetromino[i][j] != 0) {
int new_x = current_x + j;
int new_y = current_y + i;
board[new_y][new_x] = current_tetromino[i][j];
}
}
}
}
void clear_rows() {
for (int i = MAX_HEIGHT - 1; i >= 0; i--) {
int is_full = 1;
for (int j = 0; j < MAX_WIDTH; j++) {
if (board[i][j] == 0) {
is_full = 0;
break;
}
}
if (is_full) {
for (int k = i; k > 0; k--) {
for (int j = 0; j < MAX_WIDTH; j++) {
board[k][j] = board[k-1][j];
}
}
score += 10;
i++;
}
}
}
void game_over() {
printf("Game Over!\n");
printf("Your score is: %d\n", score);
}
int main() {
srand(time(NULL));
new_tetromino();
while (1) {
draw_board();
if (check_collision(current_x, current_y + 1)) {
update_board();
clear_rows();
if (current_y == 0) {
game_over();
return 0;
}
new_tetromino();
} else {
current_y++;
}
if (_kbhit()) {
int ch = _getch();
if (ch == 'a' && !check_collision(current_x - 1, current_y)) {
current_x--;
}
if (ch == 'd' && !check_collision(current_x + 1, current_y)) {
current_x++;
}
if (ch == 's' && !check_collision(current_x, current_y + 1)) {
current_y++;
}
if (ch == 'w') {
int new_tetromino[4][4];
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
new_tetromino[i][j] = current_tetromino[3-j][i];
}
}
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
current_tetromino[i][j] = new_tetromino[i][j];
}
}
if (check_collision(current_x, current_y)) {
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
new_tetromino[i][j] = current_tetromino[3-j][i];
}
}
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
current_tetromino[i][j] = new_tetromino[i][j];
}
}
}
}
if (ch == 'q') {
return 0;
}
}
Sleep(100);
}
return 0;
}
```
该示例代码使用了Windows API来实现游戏画面的显示和操作,同时使用了一个10x20的方格作为游戏场地,并通过一个二维数组来保存方块的状态。游戏中使用了随机生成的七种方块,每种方块由一个4x4的数组表示。游戏中的主要逻辑通过循环来实现,包括方块的下落、移动和旋转,以及方块堆的状态判断和更新。游戏中还实现了分数的计算和显示,以及游戏结束时的处理。
阅读全文
相关推荐
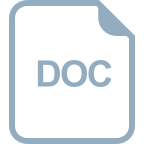
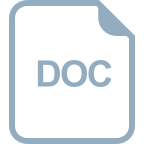
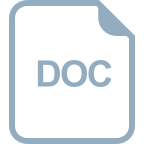
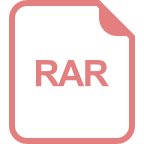
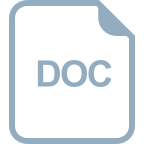
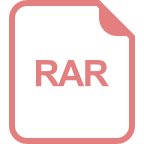
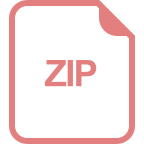
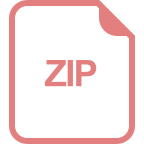
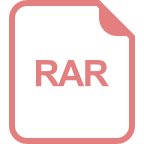
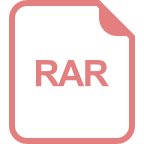
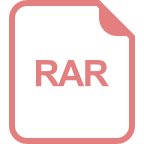
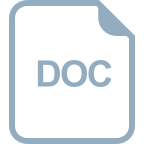
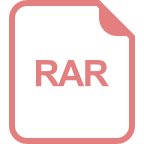
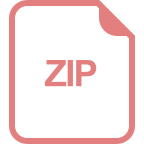
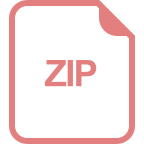
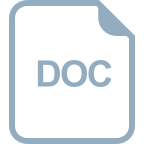


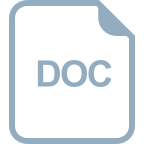