react 子组件向父组件传值
时间: 2023-07-01 19:05:14 浏览: 130
在 React 中,子组件向父组件传递值需要使用回调函数。具体步骤如下:
1. 在父组件中定义一个处理子组件传递值的函数,并将其作为 props 传递给子组件。
```javascript
class ParentComponent extends React.Component {
constructor(props) {
super(props);
this.state = {
value: ""
};
}
handleChildValue = (value) => {
this.setState({
value: value
});
}
render() {
return (
<div>
<ChildComponent onValueChange={this.handleChildValue}/>
<p>{this.state.value}</p>
</div>
);
}
}
```
2. 在子组件中触发父组件传递的回调函数,并将需要传递的值作为参数传递给它。
```javascript
class ChildComponent extends React.Component {
constructor(props) {
super(props);
this.state = {
inputValue: ""
};
}
handleChange = (event) => {
this.setState({
inputValue: event.target.value
});
this.props.onValueChange(event.target.value);
}
render() {
return (
<div>
<input type="text" value={this.state.inputValue} onChange={this.handleChange}/>
</div>
);
}
}
```
在子组件中,每当输入框的值发生变化时,就会触发 handleChange 函数,该函数会同时更新子组件的状态和触发父组件传递的回调函数。父组件接收到子组件传递的值后,就会更新自己的状态并重新渲染。这样就完成了子组件向父组件传递值的过程。
阅读全文
相关推荐
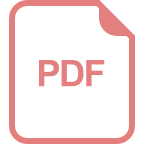
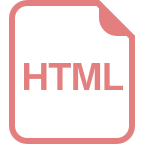










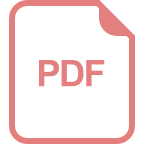



