java编写soap接口
时间: 2023-12-12 12:03:27 浏览: 136
SOAP(简易对象访问协议)是一种基于XML的协议,用于在Web应用程序之间进行通信。在Java中,可以使用Java API for XML Web Services(JAX-WS)来编写SOAP接口。
以下是编写SOAP接口的步骤:
1.创建一个Java接口,用于定义SOAP方法。
```java
package com.example.soap;
import javax.jws.WebMethod;
import javax.jws.WebService;
@WebService
public interface HelloWorld {
@WebMethod
String sayHello(String name);
}
```
2.创建一个Java类,实现上面定义的接口。
```java
package com.example.soap;
import javax.jws.WebService;
@WebService(endpointInterface = "com.example.soap.HelloWorld")
public class HelloWorldImpl implements HelloWorld {
@Override
public String sayHello(String name) {
return "Hello " + name + "!";
}
}
```
3.使用JAX-WS创建一个终端,将上面创建的实现类发布为SOAP服务。
```java
package com.example.soap;
import javax.xml.ws.Endpoint;
public class HelloWorldPublisher {
public static void main(String[] args) {
Endpoint.publish("http://localhost:8080/helloWorld", new HelloWorldImpl());
}
}
```
4.使用SOAP客户端来调用上面发布的服务。
```java
package com.example.soap;
import javax.xml.namespace.QName;
import javax.xml.ws.Service;
import java.net.URL;
public class HelloWorldClient {
public static void main(String[] args) throws Exception {
URL url = new URL("http://localhost:8080/helloWorld?wsdl");
QName qname = new QName("http://soap.example.com/", "HelloWorldImplService");
Service service = Service.create(url, qname);
HelloWorld hello = service.getPort(HelloWorld.class);
String result = hello.sayHello("World");
System.out.println(result);
}
}
```
以上就是使用Java编写SOAP接口的基本步骤。您可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
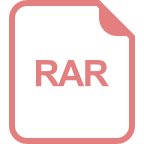
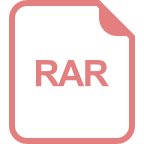
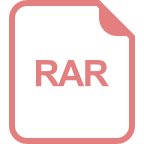
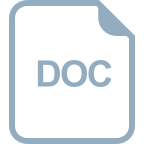



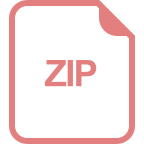
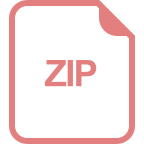
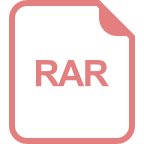
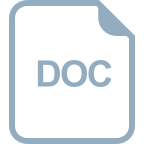
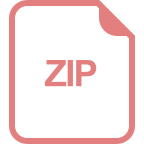
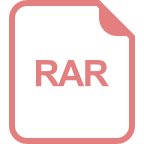
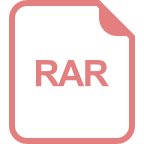
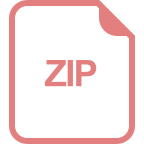
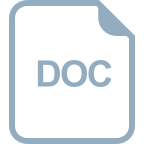
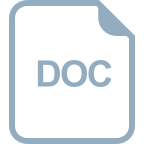