actionscript gcm加密 java解密的代码怎么写
时间: 2024-04-09 09:33:17 浏览: 50
以下是使用 ActionScript 进行 GCM 加密,并使用 Java 进行解密的代码示例:
在 ActionScript 中进行 GCM 加密:
```actionscript
import com.hurlant.crypto.symmetric.AESKey;
import com.hurlant.crypto.symmetric.GCMMode;
import com.hurlant.util.Hex;
// 加密方法
function encryptWithGCM(key:ByteArray, plaintext:String):String {
var key:ByteArray = Hex.toArray(Hex.fromString(key));
var data:ByteArray = Hex.toArray(Hex.fromString(plaintext));
var aes:AESKey = new AESKey(key);
var mode:GCMMode = new GCMMode(aes);
mode.IV = generateIV(); // 生成随机的 IV,用于加密
mode.encrypt(data); // 执行加密
var ciphertext:String = Hex.fromArray(mode.cipher);
return ciphertext;
}
// 生成随机的 IV
function generateIV():ByteArray {
var iv:ByteArray = new ByteArray();
iv.length = 16;
new Random().nextBytes(iv); // 随机生成 16 字节的 IV
return iv;
}
```
在 Java 中进行 GCM 解密:
```java
import javax.crypto.Cipher;
import javax.crypto.spec.GCMParameterSpec;
import javax.crypto.spec.SecretKeySpec;
import java.security.SecureRandom;
// 解密方法
public static String decryptWithGCM(String key, String ciphertext) throws Exception {
byte[] keyBytes = Hex.decode(key);
byte[] cipherBytes = Hex.decode(ciphertext);
SecretKeySpec secretKey = new SecretKeySpec(keyBytes, "AES");
Cipher cipher = Cipher.getInstance("AES/GCM/NoPadding");
byte[] iv = generateIV(); // 生成随机的 IV,用于解密
GCMParameterSpec gcmParameterSpec = new GCMParameterSpec(128, iv);
cipher.init(Cipher.DECRYPT_MODE, secretKey, gcmParameterSpec);
byte[] decryptedBytes = cipher.doFinal(cipherBytes);
String plaintext = new String(decryptedBytes);
return plaintext;
}
// 生成随机的 IV
public static byte[] generateIV() {
SecureRandom secureRandom = new SecureRandom();
byte[] iv = new byte[12]; // GCM 模式需要 12 字节的 IV
secureRandom.nextBytes(iv); // 随机生成 12 字节的 IV
return iv;
}
```
请注意,以上代码仅为示例,实际使用时需根据具体的需求进行适当修改和调整。此外,还需导入相关的库和类文件,以便正确执行加密和解密操作。
阅读全文
相关推荐

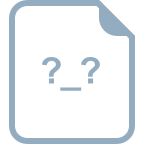
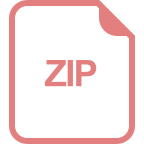

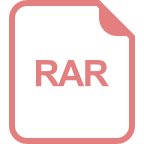

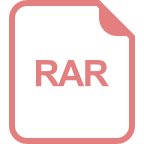
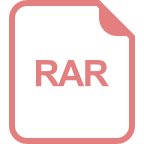


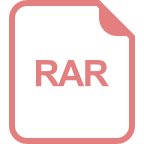
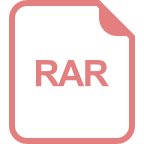
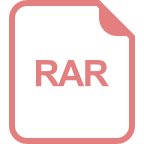