Android studio 中如何创建一个service并调用它的compara函数来比较两个数的大小,给我解决的代码
时间: 2024-03-06 09:46:25 浏览: 13
好的,以下是在 Android Studio 中创建一个 Service 并实现其中的 `compare` 函数来比较两个数字大小的示例代码:
1. 首先,在 Android Studio 中创建一个新的 Service:
- 在 Android Studio 的项目视图中,右键点击您的应用程序模块,选择 `New` -> `Service` -> `Service (Android)`
- 在弹出的对话框中,输入 Service 的名称,比如 `MyService`,并选择 `Empty Service` 模板
2. 在 `MyService` 类中实现 `compare` 函数:
```java
public class MyService extends Service {
public MyService() {
}
@Override
public IBinder onBind(Intent intent) {
// TODO: Return the communication channel to the service.
throw new UnsupportedOperationException("Not yet implemented");
}
public int compare(int a, int b) {
if (a > b) {
return 1;
} else if (a < b) {
return -1;
} else {
return 0;
}
}
}
```
3. 在您的应用程序中调用 `MyService` 的 `compare` 函数:
```java
// 创建一个 Intent 对象,用于启动 MyService
Intent intent = new Intent(this, MyService.class);
// 绑定 MyService
bindService(intent, new ServiceConnection() {
@Override
public void onServiceConnected(ComponentName componentName, IBinder iBinder) {
// 获取 MyService 的 Binder 对象
MyService.MyBinder binder = (MyService.MyBinder) iBinder;
// 调用 MyService 的 compare 函数比较两个数字的大小
int result = binder.getService().compare(10, 20);
// 输出比较结果
Log.d(TAG, "compare result: " + result);
}
@Override
public void onServiceDisconnected(ComponentName componentName) {
}
}, BIND_AUTO_CREATE);
```
在上面的代码中,我们首先创建了一个 Intent 对象,用于启动 `MyService`。然后,我们调用 `bindService()` 函数,将 Intent 对象和一个 `ServiceConnection` 对象作为参数传递给它。`bindService()` 函数用于将应用程序和 `MyService` 绑定在一起,这样我们就可以调用 `MyService` 中的函数了。
在 `ServiceConnection` 的 `onServiceConnected()` 回调函数中,我们获取了 `MyService` 的 Binder 对象,并调用了 `compare` 函数来比较两个数字的大小。最后,我们输出了比较结果。
需要注意的是,为了能够调用 `MyService` 中的函数,我们需要在 `MyService` 类中实现一个 `Binder` 对象。具体做法是,在 `MyService` 类中创建一个内部类 `MyBinder`,然后在 `onBind()` 函数中返回它的实例。这样,我们就可以通过 `MyBinder` 对象来调用 `MyService` 中的函数了。
相关推荐
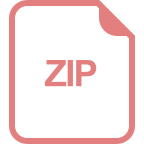
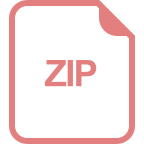
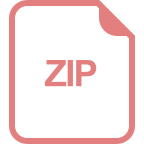




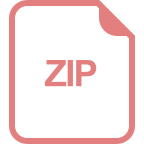
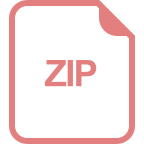
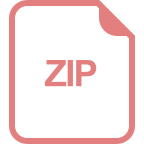
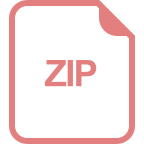
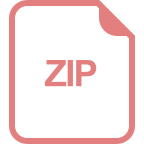
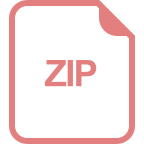