写一段代码简单实现以上功能
时间: 2024-03-01 14:52:17 浏览: 73
以下是一个简单的 Python 代码实现对比学习框架对点云数据进行编码和匹配的功能:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
# 定义特征提取器模型
class FeatureExtractor(nn.Module):
def __init__(self):
super(FeatureExtractor, self).__init__()
self.conv1 = nn.Conv1d(3, 64, 1)
self.conv2 = nn.Conv1d(64, 128, 1)
self.conv3 = nn.Conv1d(128, 256, 1)
self.conv4 = nn.Conv1d(256, 512, 1)
self.fc = nn.Linear(512, 256)
def forward(self, x):
x = F.relu(self.conv1(x))
x = F.relu(self.conv2(x))
x = F.relu(self.conv3(x))
x = F.relu(self.conv4(x))
x = torch.max(x, 2)[0]
x = self.fc(x)
return x
# 定义对比学习模型
class ContrastiveLearning(nn.Module):
def __init__(self):
super(ContrastiveLearning, self).__init__()
self.encoder = FeatureExtractor()
def forward(self, x1, x2):
# 编码输入点云数据
f1 = self.encoder(x1)
f2 = self.encoder(x2)
# 计算对比学习损失
sims = F.cosine_similarity(f1, f2, dim=1)
loss = torch.mean(1 - sims)
return loss
# 测试代码
x = torch.randn(16, 3, 1024)
x1 = x.clone()
x2 = x.clone()
x2[:, :, :512] += 0.1 # 对关键视图进行一定程度的变换
model = ContrastiveLearning()
loss = model(x1, x2)
print(loss)
```
这个代码实现了一个简单的对比学习框架,包括特征提取器和对比学习模型。我们首先定义了一个特征提取器模型,它由多个卷积层和全连接层组成,用于编码输入点云数据。然后我们定义了一个对比学习模型,它使用特征提取器对两个视图的点云数据进行编码,并计算它们在特征空间中的相似性。最后我们用两个相似但不完全相同的点云数据测试了一下模型,输出了计算得到的对比学习损失。
阅读全文
相关推荐



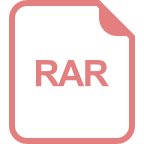
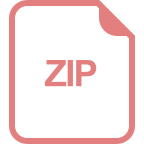




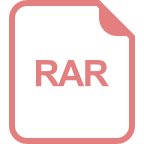








