用Java编写一个倒三角形
时间: 2023-08-21 12:01:38 浏览: 146
以下是用 Java 编写的倒三角形代码:
```java
public class InvertedTriangle {
public static void main(String[] args) {
int rows = 5;
for (int i = rows; i >= 1; i--) {
for (int j = 1; j <= i; j++) {
System.out.print("* ");
}
System.out.println();
}
}
}
```
输出结果如下:
```
* * * * *
* * * *
* * *
* *
*
```
其中,`rows` 变量表示倒三角形的行数,通过两层循环输出星号,外层循环控制行数,内层循环控制每行输出的星号个数。由于是倒三角形,所以外层循环从 `rows` 开始倒序循环,而内层循环则从 `1` 开始正序循环。每行输出完毕后需要换行,使用 `System.out.println()` 实现。
相关问题
用java编写一个求三角形面积的代码
在Java中,计算三角形面积的一个简单方法是基于海伦公式或者基础边长乘以对应高的方法。这里给出两种常见的做法:
1. **使用基础边长和高**:
```java
public class TriangleArea {
public static double calculateTriangleArea(double base, double height) {
return 0.5 * base * height;
}
public static void main(String[] args) {
double base = 5.0; // 三角形底边长度
double height = 3.0; // 三角形对应的高度
double area = calculateTriangleArea(base, height);
System.out.println("The area of the triangle is " + area + " square units.");
}
}
```
2. **使用海伦公式** (适用于已知三边的情况),对于任意三角形,可以先计算半周长,然后应用公式`area = sqrt[p*(p-a)*(p-b)*(p-c)]`,其中a、b、c是三角形的三个边长,p是半周长((a+b+c)/2):
```java
import java.util.Scanner;
public class TriangleAreaHeron {
public static double calculateHeronArea(double a, double b, double c) {
double semiperimeter = (a + b + c) / 2;
double area = Math.sqrt(semiperimeter * (semiperimeter - a) * (semiperimeter - b) * (semiperimeter - c));
return area;
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter side a: ");
double a = scanner.nextDouble();
System.out.print("Enter side b: ");
double b = scanner.nextDouble();
System.out.print("Enter side c: ");
double c = scanner.nextDouble();
double area = calculateHeronArea(a, b, c);
System.out.println("The area of the triangle is " + area + " square units.");
}
}
```
用java编写一个三角形
以下是使用Java编写的三角形程序:
```
public class Triangle {
public static void main(String[] args) {
int rows = 5;
for (int i = 1; i <= rows; i++) {
for (int j = 1; j <= i; j++) {
System.out.print("* ");
}
System.out.println();
}
}
}
```
该程序将输出一个五行的三角形,如下所示:
```
*
* *
* * *
* * * *
* * * * *
```
阅读全文
相关推荐
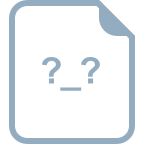
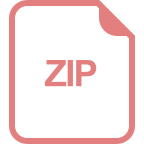













