python读写txt检测文件是否存在
时间: 2023-08-21 18:04:34 浏览: 53
你可以使用Python的`os`模块来检测文件是否存在。具体的方法是使用`os.path.isfile()`函数来判断文件是否存在。下面是一个例子:
```python
import os
filename = 'example.txt'
if os.path.isfile(filename):
print("文件存在")
else:
print("文件不存在")
```
在上面的例子中,我们首先定义了一个文件名`example.txt`,然后使用`os.path.isfile()`函数来判断文件是否存在。如果文件存在,就输出"文件存在";如果文件不存在,就输出"文件不存在"。
另外,如果你想要读取或写入文本文件,你可以使用Python的内置函数`open()`来打开文件。例如,你可以使用以下代码来读取文件内容:
```python
filename = 'example.txt'
if os.path.isfile(filename):
with open(filename, 'r') as file:
content = file.read()
print(content)
else:
print("文件不存在")
```
在上面的代码中,我们使用`open()`函数打开文件,并指定打开模式为'r'(只读)。然后使用`read()`方法读取文件内容,并打印出来。记得使用完文件后,要使用`close()`方法关闭文件。但是更好的做法是使用上下文管理器`with open()`来自动关闭文件。
相关问题
python读取txt文件显示编码错误怎么办
当 Python 读取 txt 文件时显示编码错误时,可以尝试以下方法:
1. 指定文件编码方式
在读取文件时,可以通过指定文件的编码方式来解决编码错误问题。例如:
```python
with open('file.txt', 'r', encoding='utf-8') as f:
# 读取文件内容
```
2. 使用 chardet 库自动检测编码
```python
import chardet
with open('file.txt', 'rb') as f:
content = f.read()
result = chardet.detect(content)
encoding = result['encoding']
with open('file.txt', 'r', encoding=encoding) as f:
# 读取文件内容
```
3. 使用 UnicodeDecodeError 错误处理
当读取文件时出现 UnicodeDecodeError 错误时,可以使用 try-except 语句来捕获该错误并进行处理。例如:
```python
try:
with open('file.txt', 'r') as f:
# 读取文件内容
except UnicodeDecodeError:
with open('file.txt', 'r', encoding='utf-8') as f:
# 读取文件内容
```
希望这些方法可以帮助你解决编码错误问题。
如何用python检查txt文件中存在乱码
在Python中,可以使用`chardet`库来检查文本文件中的编码。下面是一个简单的示例:
```python
import chardet
with open('example.txt', 'rb') as f:
data = f.read()
result = chardet.detect(data)
if result['encoding'] == 'utf-8':
print('文件编码正确')
else:
print('文件编码错误,编码为', result['encoding'])
```
上面的代码首先打开一个文本文件,读取其中的内容,然后使用`chardet.detect()`函数检测文件的编码。如果编码为UTF-8,则输出“文件编码正确”,否则输出“文件编码错误,编码为xxx”。
相关推荐
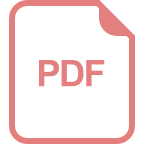
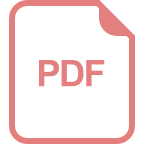
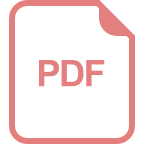












