怎么封装一个可以全局使用的localStorage
时间: 2024-05-05 11:19:14 浏览: 17
存储操作类?
要封装一个可以全局使用的localStorage存储操作类,可以按照以下步骤进行:
1. 创建一个Storage类,用来封装localStorage的读写操作方法。
2. 通过ES6的class方式定义Storage类,可以包含如下方法:
- set(key: string, value: any): void //存储某个键值对
- get(key: string): any //获取某个键对应的值
- remove(key: string): void //移除某个键值对
- clear(): void //清除所有的键值对
3. 在Storage类中,可以通过判断浏览器是否支持localStorage来决定使用哪种存储方式,如localStorage或cookie等。
4. 定义一个全局变量,用来存储Storage实例,可以在需要使用localStorage的时候,直接调用全局变量中的Storage实例即可。
5. 最后,在页面加载的时候,初始化全局变量中的Storage实例,并向全局下挂载Storage对象,以便在任何地方都可以使用。
示例代码如下:
```javascript
class Storage {
constructor() {
this.store = window.localStorage || null;
}
set(key, value) {
if (this.store) {
this.store.setItem(key, JSON.stringify(value));
}
}
get(key) {
if (this.store) {
const value = this.store.getItem(key);
return JSON.parse(value);
}
}
remove(key) {
if (this.store) {
this.store.removeItem(key);
}
}
clear() {
if (this.store) {
this.store.clear();
}
}
}
const storage = new Storage();
window.Storage = storage;
```
注:上述代码中,使用JSON.stringify()和JSON.parse()方法将值转为JSON字符串和对象,以便能够储存和读取对象类型的数据。
相关推荐
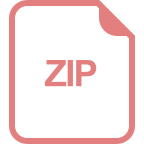
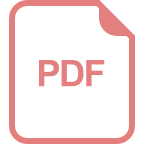










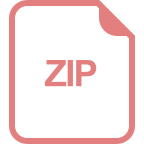