帮我用C#写一个程序,一个RV减速机,针齿中心圆为52.5,针齿半径为2.25,针齿轮数为40,摆线轮齿数为39,偏心距为1,生成摆线轮外摆线的坐标点,并保存txt文件
时间: 2024-05-16 18:17:39 浏览: 7
好的,我可以帮你写这个程序。
首先,我们需要计算摆线轮的齿数和模数:
```csharp
int pinionTeeth = 39; // 摆线轮齿数
int gearTeeth = 40; // 针齿轮齿数
double module = 2 * 2.25 / (pinionTeeth + gearTeeth); // 模数
```
然后,我们需要计算摆线轮的基圆半径和分度圆半径:
```csharp
double pinionBaseRadius = (pinionTeeth + 2) * module / 2; // 基圆半径
double pinionPitchRadius = pinionTeeth * module / 2; // 分度圆半径
```
接着,我们需要生成摆线轮的外摆线坐标点:
```csharp
double eccentricity = 1; // 偏心距
double thetaStep = 2 * Math.PI / 1000; // 步长
List<PointF> points = new List<PointF>(); // 存储坐标点的列表
for (double theta = 0; theta <= 2 * Math.PI; theta += thetaStep)
{
double x = (pinionBaseRadius + eccentricity) * Math.Cos(theta) - eccentricity * Math.Cos((pinionBaseRadius + eccentricity) / pinionPitchRadius * theta);
double y = (pinionBaseRadius + eccentricity) * Math.Sin(theta) - eccentricity * Math.Sin((pinionBaseRadius + eccentricity) / pinionPitchRadius * theta);
points.Add(new PointF((float)x, (float)y));
}
```
最后,我们将生成的坐标点保存到txt文件中:
```csharp
string filePath = "points.txt";
using (StreamWriter writer = File.CreateText(filePath))
{
foreach (PointF point in points)
{
writer.WriteLine(point.X + "\t" + point.Y);
}
}
```
完整代码如下:
```csharp
using System;
using System.Collections.Generic;
using System.Drawing;
using System.IO;
class Program
{
static void Main(string[] args)
{
int pinionTeeth = 39; // 摆线轮齿数
int gearTeeth = 40; // 针齿轮齿数
double module = 2 * 2.25 / (pinionTeeth + gearTeeth); // 模数
double pinionBaseRadius = (pinionTeeth + 2) * module / 2; // 基圆半径
double pinionPitchRadius = pinionTeeth * module / 2; // 分度圆半径
double eccentricity = 1; // 偏心距
double thetaStep = 2 * Math.PI / 1000; // 步长
List<PointF> points = new List<PointF>(); // 存储坐标点的列表
for (double theta = 0; theta <= 2 * Math.PI; theta += thetaStep)
{
double x = (pinionBaseRadius + eccentricity) * Math.Cos(theta) - eccentricity * Math.Cos((pinionBaseRadius + eccentricity) / pinionPitchRadius * theta);
double y = (pinionBaseRadius + eccentricity) * Math.Sin(theta) - eccentricity * Math.Sin((pinionBaseRadius + eccentricity) / pinionPitchRadius * theta);
points.Add(new PointF((float)x, (float)y));
}
string filePath = "points.txt";
using (StreamWriter writer = File.CreateText(filePath))
{
foreach (PointF point in points)
{
writer.WriteLine(point.X + "\t" + point.Y);
}
}
}
}
```
你可以将以上代码复制到Visual Studio中运行,即可生成txt文件。
相关推荐
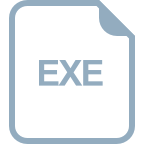














