JAVA1.8标准 扫描指定目录及其子目录下的所有文件,将所有文件的完整路径、大小以JSON的数据格式写入到指定路径下的txt文件中
时间: 2024-05-04 07:16:40 浏览: 91
可以使用Java的File类和递归方法来扫描指定目录及其子目录下的所有文件,并使用JSONObject类将文件的完整路径和大小封装到JSON对象中。然后可以将JSON对象写入到指定路径下的txt文件中,可以使用Java的FileWriter和BufferedWriter类实现。以下是示例代码:
```
import java.io.*;
import java.nio.file.*;
import org.json.*;
public class FileScanner {
public static void scanFiles(String directoryPath, String outputPath) {
try {
JSONObject json = new JSONObject();
scanFiles(directoryPath, json);
FileWriter writer = new FileWriter(outputPath);
BufferedWriter bufferedWriter = new BufferedWriter(writer);
bufferedWriter.write(json.toString());
bufferedWriter.close();
} catch (JSONException | IOException e) {
e.printStackTrace();
}
}
private static void scanFiles(String directoryPath, JSONObject json) throws IOException {
File[] files = new File(directoryPath).listFiles();
for (File file : files) {
if (file.isFile()) {
json.put(file.getAbsolutePath(), file.length());
} else if (file.isDirectory()) {
scanFiles(file.getAbsolutePath(), json);
}
}
}
public static void main(String[] args) {
String directoryPath = "path/to/directory";
String outputPath = "path/to/output.txt";
scanFiles(directoryPath, outputPath);
}
}
```
在程序中将文件的完整路径和大小封装到JSON对象中,然后调用JSONObject的toString方法将其转换为JSON字符串,并将其写入到指定路径下的txt文件中。您可以将"path/to/directory"和"path/to/output.txt"替换为您实际的目录和输出路径。
阅读全文
相关推荐
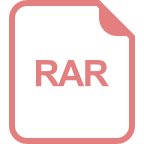
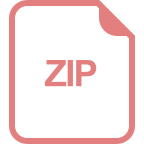
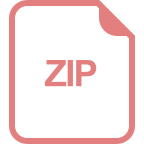
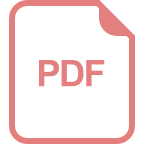
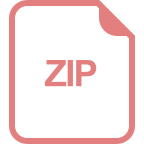
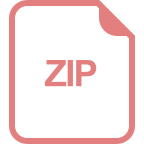
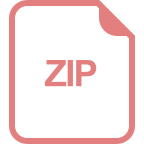
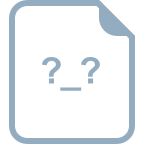
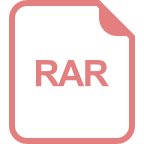
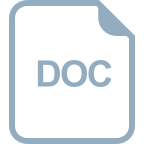
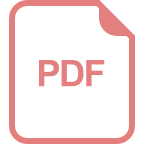
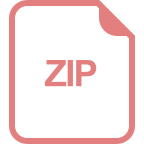
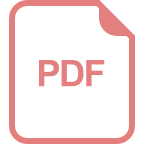
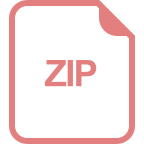
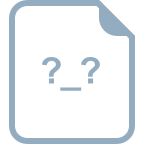