C++编译有一学生成绩表,包括学号、姓名、3门课程成绩。请实现如下删除功能:输入一个学生的学号,删除该学生的所有信息。
时间: 2024-06-08 12:09:20 浏览: 10
以下是一个简单的实现:
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
struct Student {
string id;
string name;
int score1;
int score2;
int score3;
};
int main() {
vector<Student> students;
while (true) {
cout << "请输入学生的学号、姓名、3门课程成绩(用空格隔开),输入exit结束输入:" << endl;
string id, name;
int score1, score2, score3;
cin >> id;
if (id == "exit") {
break;
}
cin >> name >> score1 >> score2 >> score3;
students.push_back({id, name, score1, score2, score3});
}
cout << "请输入要删除的学生的学号:" << endl;
string delete_id;
cin >> delete_id;
bool found = false;
for (int i = 0; i < students.size(); i++) {
if (students[i].id == delete_id) {
students.erase(students.begin() + i);
found = true;
break;
}
}
if (found) {
cout << "删除成功!" << endl;
} else {
cout << "没有找到该学生的信息。" << endl;
}
cout << "学生信息如下:" << endl;
for (const auto& student : students) {
cout << student.id << " " << student.name << " " << student.score1 << " " << student.score2 << " " << student.score3 << endl;
}
return 0;
}
```
在这个程序中,我们使用了一个 `vector` 来存储多个学生的信息。在输入学生信息时,我们使用 `while` 循环不断读入学生信息,并将每个学生的信息存储在一个 `Student` 结构体中,然后将该结构体保存在 `students` 向量中。
当用户输入要删除的学生的学号后,我们遍历 `students` 向量,找到该学生的信息并将其删除。我们使用一个布尔变量 `found` 来表示是否找到了该学生的信息。如果找到了,我们输出删除信息成功的提示;否则,输出没有找到该学生的信息的提示。
最后,我们遍历 `students` 向量,输出所有学生的信息。
相关推荐
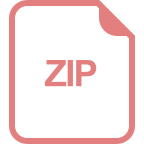
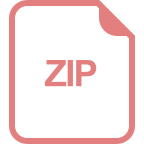
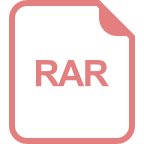














