Java生成netty服务器启动程序
时间: 2023-08-14 20:04:55 浏览: 99
下面是一个简单的示例代码,用于生成一个基本的Netty服务器启动器:
```java
import io.netty.bootstrap.ServerBootstrap;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelOption;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioServerSocketChannel;
public class NettyServer {
private final int port;
public NettyServer(int port) {
this.port = port;
}
public void run() throws InterruptedException {
// 创建主线程组
NioEventLoopGroup bossGroup = new NioEventLoopGroup();
// 创建工作线程组
NioEventLoopGroup workerGroup = new NioEventLoopGroup();
try {
// 创建服务器启动器
ServerBootstrap serverBootstrap = new ServerBootstrap();
serverBootstrap.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) throws Exception {
// 添加自定义的ChannelHandler
ch.pipeline().addLast(new NettyServerHandler());
}
})
.option(ChannelOption.SO_BACKLOG, 128)
.childOption(ChannelOption.SO_KEEPALIVE, true);
// 绑定端口并启动服务器
ChannelFuture channelFuture = serverBootstrap.bind(port).sync();
// 等待服务器关闭
channelFuture.channel().closeFuture().sync();
} finally {
// 关闭线程组
bossGroup.shutdownGracefully();
workerGroup.shutdownGracefully();
}
}
public static void main(String[] args) throws InterruptedException {
int port = 8080;
NettyServer server = new NettyServer(port);
server.run();
}
}
```
在上面的示例代码中,我们首先创建了两个NioEventLoopGroup线程组,一个用于处理客户端连接,另一个用于处理客户端请求。然后,我们创建了一个ServerBootstrap实例,并将它们绑定在一起。接下来,我们设置了服务器通道选项和子通道选项,以及自定义的ChannelHandler。最后,我们绑定了服务器端口并启动服务器。
阅读全文
相关推荐
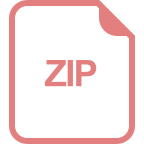
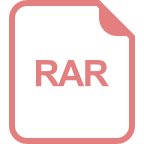


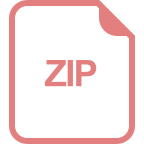
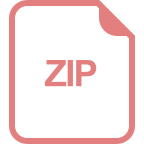
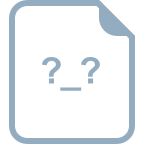
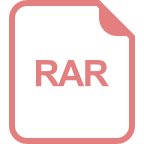
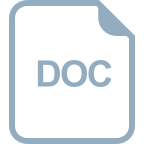
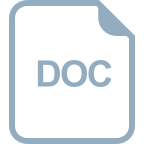
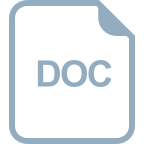
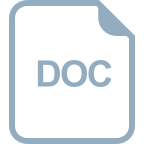
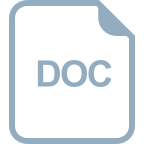
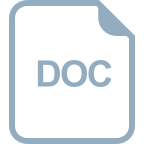
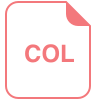


