udp原始套接字icmp
时间: 2023-07-27 16:05:43 浏览: 53
UDP是一种无连接的传输协议,它不保证数据包的可靠性和顺序性。而ICMP是一种网络协议,它主要用于网络故障排查、错误报告和路由器之间的通信。在使用UDP协议时,我们可以使用原始套接字来发送和接收ICMP消息。
在Linux系统中,我们可以使用socket()函数来创建一个原始套接字,指定协议族为AF_INET(IPv4)或AF_INET6(IPv6),协议类型为SOCK_RAW,协议号为IPPROTO_ICMP。然后我们可以使用sendto()函数来发送UDP数据包,并使用recvfrom()函数来接收ICMP消息。
以下是一个简单的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <netinet/ip.h>
#include <netinet/ip_icmp.h>
#define BUFSIZE 1024
int main(int argc, char *argv[]) {
int sockfd;
struct sockaddr_in addr;
char buffer[BUFSIZE];
// 创建原始套接字
sockfd = socket(AF_INET, SOCK_RAW, IPPROTO_ICMP);
if (sockfd < 0) {
perror("socket");
exit(EXIT_FAILURE);
}
// 设置地址结构体
memset(&addr, 0, sizeof(addr));
addr.sin_family = AF_INET;
addr.sin_addr.s_addr = inet_addr("127.0.0.1");
// 发送UDP数据包
strcpy(buffer, "Hello, UDP!");
if (sendto(sockfd, buffer, strlen(buffer), 0, (struct sockaddr *)&addr, sizeof(addr)) < 0) {
perror("sendto");
exit(EXIT_FAILURE);
}
// 接收ICMP消息
if (recvfrom(sockfd, buffer, BUFSIZE, 0, NULL, NULL) < 0) {
perror("recvfrom");
exit(EXIT_FAILURE);
}
struct iphdr *iph = (struct iphdr *)buffer;
struct icmphdr *icmph = (struct icmphdr *)(buffer + (iph->ihl * 4));
printf("Received ICMP packet from %s\n", inet_ntoa(addr.sin_addr));
printf("Type: %d\n", icmph->type);
printf("Code: %d\n", icmph->code);
close(sockfd);
return 0;
}
```
在上面的示例代码中,我们创建了一个原始套接字,并指定协议为ICMP。然后我们使用sendto()函数发送了一个UDP数据包,目标地址为127.0.0.1。接着,我们使用recvfrom()函数接收ICMP消息,并解析出其中的类型和代码。
需要注意的是,使用原始套接字需要具有管理员权限。此外,使用原始套接字需要特殊的权限,因此需要小心使用。
相关推荐
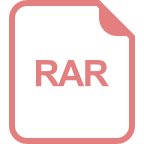
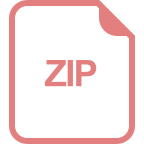
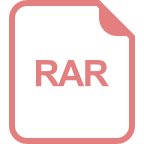










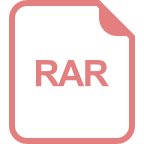
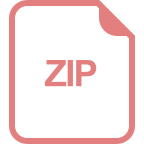
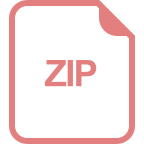
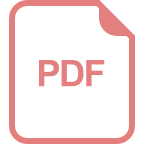