使用Spring Boot和Vue.js构建一个简单的前后端交互应用,在后端从数据库中取出用户数据,在前端显示取出的用户数据,并且可以对取出的数据进行操作,如增删查改,操作后的数据存入数据库中
时间: 2024-04-03 11:30:53 浏览: 136
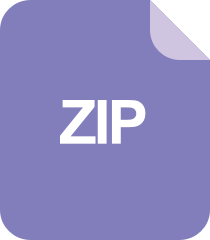
Springboot+vue商城
好的,这是一个非常常见的应用场景。我可以为您提供一些简单的指导来实现这个应用。
首先,您需要创建一个Spring Boot应用程序,并且使用Vue.js创建前端应用程序。在后端,您需要使用Spring Data JPA来管理数据。在前端,您可以使用Vue.js和axios来与后端进行通信。
以下是一些步骤,可以帮助您完成这个应用程序:
1. 创建一个Spring Boot应用程序
您可以使用Spring Initializr(https://start.spring.io/)来创建一个新的Spring Boot应用程序。选择您喜欢的构建工具(Maven或Gradle),选择Spring Boot版本,并选择一些必要的依赖项(如Spring Web和Spring Data JPA)。
2. 创建一个数据模型
在后端,您需要定义一个数据模型来表示用户数据。您可以使用JPA注解来定义一个实体类,并且定义与数据库表的映射关系。例如:
```java
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false)
private String name;
@Column(nullable = false)
private Integer age;
// getters and setters
}
```
3. 创建一个数据访问层
在后端,您需要创建一个数据访问层来管理数据。您可以使用Spring Data JPA来简化数据访问。例如:
```java
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
}
```
4. 创建一个控制器
在后端,您需要创建一个控制器来处理HTTP请求。您可以使用Spring MVC来创建控制器。例如:
```java
@RestController
@RequestMapping("/api/users")
public class UserController {
@Autowired
private UserRepository userRepository;
@GetMapping
public List<User> getUsers() {
return userRepository.findAll();
}
@PostMapping
public User createUser(@RequestBody User user) {
return userRepository.save(user);
}
@PutMapping("/{id}")
public User updateUser(@PathVariable Long id, @RequestBody User user) {
user.setId(id);
return userRepository.save(user);
}
@DeleteMapping("/{id}")
public void deleteUser(@PathVariable Long id) {
userRepository.deleteById(id);
}
}
```
5. 创建一个前端应用程序
在前端,您需要使用Vue.js创建一个应用程序。您可以使用Vue CLI(https://cli.vuejs.org/)来创建一个新的应用程序。
6. 创建一个用户列表组件
在前端,您需要创建一个组件来显示用户列表。您可以使用axios来从后端获取用户数据,并使用Vue.js来显示数据。例如:
```html
<template>
<div>
<table>
<thead>
<tr>
<th>Id</th>
<th>Name</th>
<th>Age</th>
<th>Actions</th>
</tr>
</thead>
<tbody>
<tr v-for="user in users" :key="user.id">
<td>{{ user.id }}</td>
<td>{{ user.name }}</td>
<td>{{ user.age }}</td>
<td>
<button @click="editUser(user)">Edit</button>
<button @click="deleteUser(user)">Delete</button>
</td>
</tr>
</tbody>
</table>
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
users: [],
};
},
methods: {
loadUsers() {
axios.get('/api/users').then((response) => {
this.users = response.data;
});
},
editUser(user) {
// TODO: Implement edit user
},
deleteUser(user) {
axios.delete(`/api/users/${user.id}`).then(() => {
this.loadUsers();
});
},
},
mounted() {
this.loadUsers();
},
};
</script>
```
7. 创建一个用户表单组件
在前端,您需要创建一个组件来添加/编辑用户。您可以使用Vue.js和axios来向后端提交数据。例如:
```html
<template>
<div>
<form>
<div>
<label>Name:</label>
<input type="text" v-model="user.name" />
</div>
<div>
<label>Age:</label>
<input type="number" v-model="user.age" />
</div>
<div>
<button type="submit" @click.prevent="saveUser">Save</button>
<button type="button" @click="cancel">Cancel</button>
</div>
</form>
</div>
</template>
<script>
import axios from 'axios';
export default {
props: ['user'],
methods: {
saveUser() {
if (this.user.id) {
axios.put(`/api/users/${this.user.id}`, this.user).then(() => {
this.$emit('saved');
});
} else {
axios.post('/api/users', this.user).then(() => {
this.$emit('saved');
});
}
},
cancel() {
this.$emit('canceled');
},
},
};
</script>
```
8. 创建一个应用程序路由
在前端,您需要创建一个路由来管理应用程序的不同页面。您可以使用Vue Router(https://router.vuejs.org/)来创建一个路由。例如:
```javascript
import Vue from 'vue';
import VueRouter from 'vue-router';
import UserList from './components/UserList.vue';
import UserForm from './components/UserForm.vue';
Vue.use(VueRouter);
const routes = [
{ path: '/', component: UserList },
{ path: '/users/new', component: UserForm },
{ path: '/users/:id/edit', component: UserForm, props: true },
];
const router = new VueRouter({
mode: 'history',
routes,
});
export default router;
```
9. 集成前端和后端
最后,您需要将前端和后端集成起来。您可以使用axios来向后端发送HTTP请求,并使用Vue.js来显示数据和处理用户交互。
现在,您应该已经了解如何使用Spring Boot和Vue.js创建一个简单的前后端交互应用程序了。当然,这只是一个简单的示例,您可以根据自己的需求进行扩展和改进。
阅读全文
相关推荐
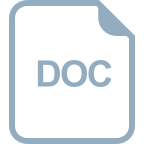
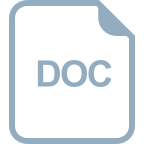
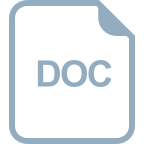


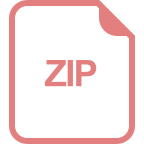
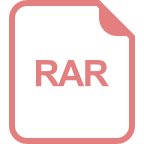
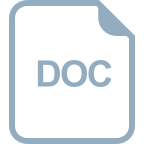
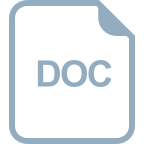
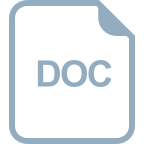
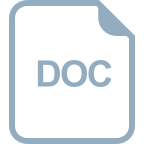
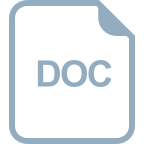
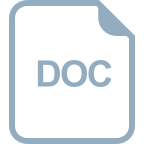
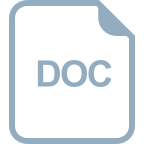
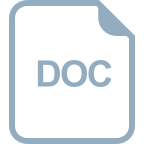


