C语言遍历文件夹下的所有文件
时间: 2023-09-12 08:10:31 浏览: 502
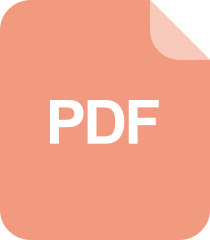
C语言 实现遍历一个文件夹的所有文件

你可以使用标准库中的 dirent.h 和 sys/stat.h,使用 opendir() 打开目录,然后使用 readdir() 循环读取目录下的所有文件和子目录,再使用 stat() 获取文件信息。具体实现可以参考以下代码:
```c
#include <stdio.h>
#include <dirent.h>
#include <sys/stat.h>
void traverse_dir(char *dir_path)
{
DIR *dir;
struct dirent *entry;
struct stat stat_buf;
if ((dir = opendir(dir_path)) == NULL) {
printf("Error: can't open directory %s\n", dir_path);
return;
}
chdir(dir_path); // 切换当前目录到遍历的目录下
while ((entry = readdir(dir)) != NULL) {
lstat(entry->d_name, &stat_buf); // 获取文件信息
if (S_ISDIR(stat_buf.st_mode)) { // 如果是子目录,递归遍历
if (strcmp(".", entry->d_name) == 0 || strcmp("..", entry->d_name) == 0)
continue;
printf("subdir: %s/\n", entry->d_name);
traverse_dir(entry->d_name);
}
else { // 如果是文件,输出文件名和大小
printf("file: %s ", entry->d_name);
printf("size: %ld bytes\n", stat_buf.st_size);
}
}
chdir(".."); // 切换回上级目录
closedir(dir);
}
int main()
{
traverse_dir("/path/to/dir"); // 遍历 /path/to/dir 目录下的所有文件和目录
return 0;
}
```
阅读全文
相关推荐
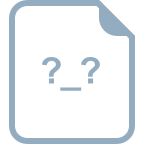
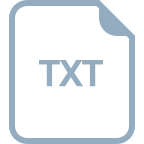
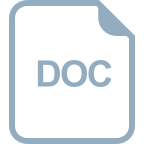
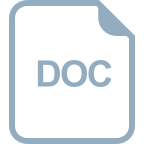

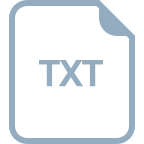
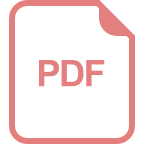
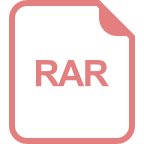
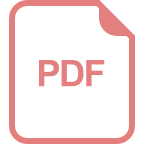
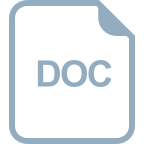
