javascript解析xml转json
时间: 2023-06-29 21:09:04 浏览: 114
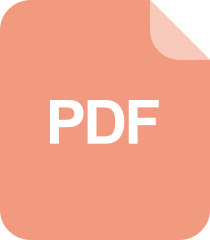
javascript解析ajax返回的xml和json格式数据实例详解
可以使用JavaScript内置的XML解析器`DOMParser`来解析XML,然后将解析结果转换为JSON格式。以下是一个示例代码:
```javascript
function xmlToJson(xml) {
// Create the DOM parser
const parser = new DOMParser();
// Parse the XML string
const xmlDoc = parser.parseFromString(xml, "text/xml");
// Get the root element of the XML document
const root = xmlDoc.documentElement;
// Convert the XML document to a JSON object
return convertToJson(root);
}
function convertToJson(node) {
const obj = {};
if (node.nodeType === 1) { // element node
if (node.attributes.length > 0) {
obj["@attributes"] = {};
for (let i = 0; i < node.attributes.length; i++) {
const attr = node.attributes.item(i);
obj["@attributes"][attr.nodeName] = attr.nodeValue;
}
}
} else if (node.nodeType === 3) { // text node
obj = node.nodeValue;
}
if (node.hasChildNodes()) {
for (let i = 0; i < node.childNodes.length; i++) {
const childNode = node.childNodes.item(i);
const nodeName = childNode.nodeName;
if (typeof(obj[nodeName]) === "undefined") {
obj[nodeName] = convertToJson(childNode);
} else {
if (typeof(obj[nodeName].push) === "undefined") {
const oldObj = obj[nodeName];
obj[nodeName] = [];
obj[nodeName].push(oldObj);
}
obj[nodeName].push(convertToJson(childNode));
}
}
}
return obj;
}
```
使用方法:
```javascript
const xmlString = "<bookstore><book category='cooking'><title lang='en'>Everyday Italian</title><author>Giada De Laurentiis</author><year>2005</year><price>30.00</price></book><book category='children'><title lang='en'>Harry Potter</title><author>J.K. Rowling</author><year>2005</year><price>29.99</price></book></bookstore>";
const json = xmlToJson(xmlString);
console.log(json);
```
输出结果:
```json
{
"bookstore": {
"@attributes": {},
"book": [
{
"@attributes": {
"category": "cooking"
},
"title": {
"@attributes": {
"lang": "en"
},
"#text": "Everyday Italian"
},
"author": {
"#text": "Giada De Laurentiis"
},
"year": {
"#text": "2005"
},
"price": {
"#text": "30.00"
}
},
{
"@attributes": {
"category": "children"
},
"title": {
"@attributes": {
"lang": "en"
},
"#text": "Harry Potter"
},
"author": {
"#text": "J.K. Rowling"
},
"year": {
"#text": "2005"
},
"price": {
"#text": "29.99"
}
}
]
}
}
```
阅读全文
相关推荐
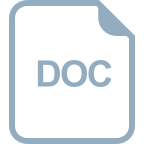
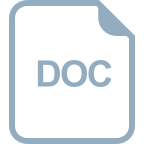
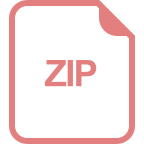
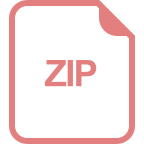
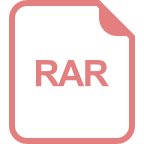
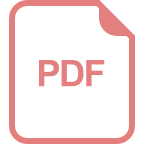
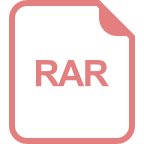
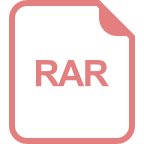
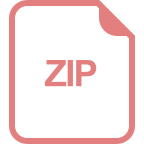
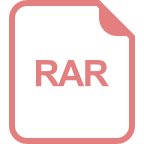
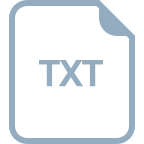
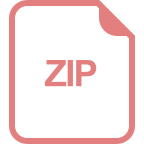
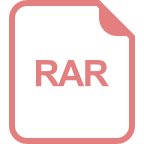
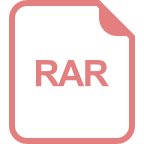
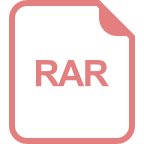
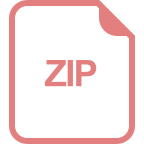
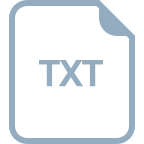
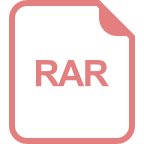