使用C++编写一个声明一个Tree(树)类,有成员ages(树龄),成员函数grow(int years)用以对ages 加上years,showage( )用以显示tree对象的ages值。在主函数中定义Tree类对
时间: 2024-05-31 08:07:02 浏览: 6
#include <stdio.h>
class Tree {
private:
int ages;
public:
Tree(int a) {
ages = a;
}
void grow(int years) {
ages += years;
}
void showage() {
printf("The age of the tree is %d years old.\n", ages);
}
};
int main() {
Tree tree(10);
tree.showage();
tree.grow(5);
tree.showage();
return 0;
}
相关问题
声明一个Tree(树)类,有成员ages(树龄),成员函数grow(int years)用以对ages 加上years,showage( )用以显示tree对象的ages值。在主函数中定义Tree类对 使用C++
++语言实现如下:
#include <iostream>
using namespace std;
class Tree {
private:
int ages;
public:
Tree(int a = 0) { ages = a; } // 构造函数
void grow(int years) { ages += years; } // 成长函数
void showage() { cout << "The age of the tree is " << ages << " years." << endl; } // 显示函数
};
int main() {
Tree oak(5); // 创建一个树龄为5年的橡树对象
oak.showage(); // 显示树龄
oak.grow(3); // 让橡树增长3年
oak.showage(); // 再次显示树龄
return 0;
}
用C++定义一个Tree(树)类,有成员ages(树龄),成员函数grow(int years)对ages加上years,ages()显示tree对象的ages的值。
好的,这是一个简单的Tree类的定义:
```c++
class Tree {
private:
int ages;
public:
Tree(int ages = 0) : ages(ages) {}
void grow(int years) {
ages += years;
}
int getAges() const {
return ages;
}
};
```
使用方法:
```c++
Tree tree(10);
tree.grow(5);
cout << "The ages of the tree is: " << tree.getAges() << endl;
```
输出结果:
```
The ages of the tree is: 15
```
相关推荐
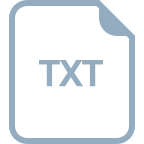
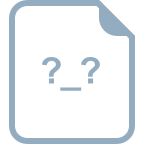
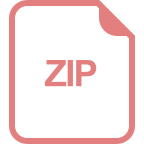












