num = int(input("输入数字:")) scores = [90,80,70,60] levels = ['A','B','C','D'] for i, s in enumerate(scores): if num >= s: print("等级为:", levels[i]) break 详细解释一下上述代码
时间: 2024-03-08 17:48:16 浏览: 13
这段代码是 Python 语言编写的一个简单的等级划分程序,根据输入的数字 num,输出对应的等级。以下是代码的详细解释:
```python
num = int(input("输入数字:")) # 获取用户输入的数字,转换为整型
scores = [90,80,70,60] # 定义分数阈值列表
levels = ['A','B','C','D'] # 定义等级列表
# 使用 for 循环遍历分数阈值列表
for i, s in enumerate(scores):
if num >= s: # 如果用户输入的数字大于等于当前阈值
print("等级为:", levels[i]) # 输出对应的等级
break # 结束循环
```
代码的执行流程如下:
1. 用户输入一个数字,该数字被转换为整型并赋值给变量 num。
2. 定义了两个列表 scores 和 levels,分别存储分数阈值和对应的等级。
3. 使用 for 循环遍历分数阈值列表 scores,并使用 enumerate 函数获取当前阈值的下标 i 和对应的分数阈值 s。
4. 在循环中,如果用户输入的数字 num 大于等于当前阈值 s,就输出对应的等级 levels[i],并使用 break 结束循环。
5. 如果用户输入的数字 num 没有大于任何一个分数阈值,程序不会输出任何内容。
例如,如果用户输入的数字是 75,程序会输出等级为:C。
相关问题
score_dict = {}while True: input_str = input().strip() if input_str == '#': break name, score = input_str.split() score = int(score) if name in score_dict: score_dict[name].append(score) else: score_dict[name] = [score]print('姓 名 平均成绩')for name, scores in score_dict: avg_score = sum(scores) / len(scores) print('{:<8} {:.2f}'.format(name, avg_score))
这段代码存在一些问题,应该修改为:
```python
score_dict = {}
while True:
input_str = input().strip()
if input_str == '#':
break
name, score = input_str.split()
score = int(score)
if name in score_dict:
score_dict[name].append(score)
else:
score_dict[name] = [score]
print('姓 名 平均成绩')
for name, scores in score_dict.items():
avg_score = sum(scores) / len(scores)
print('{:<8} {:.2f}'.format(name, avg_score))
```
主要修改包括:
- 对于 `for name, scores in score_dict`,应该改为 `for name, scores in score_dict.items()`,因为字典类型需要使用 `items()` 方法来遍历。
- 对于计算平均成绩,应该在遍历时进行,而不是在输入时计算。
n=int(input()) for i in range(n): scores=map(int,input().split()) print(max(scores))优化缩短运行时间
你可以使用列表解析式来取代 for 循环,同时使用内置函数 map() 和 max(),将代码简化为一行:
```python
print(*[max(map(int, input().split())) for _ in range(int(input()))])
```
这样可以减少循环次数和代码行数,从而优化缩短运行时间。
相关推荐
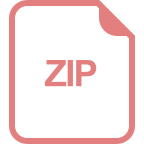
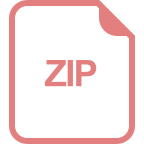
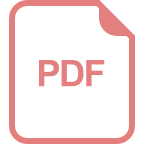












