② 使用linux操作系统设计一个C语言程序实现以下功能: a.屏幕上显示功能菜单: 1:get a message queue * 2:sending message * 3:receiving message * 3:remove a message queue * 0:Exit * b.接收用户的选择 c.根据用户的选择,创建消息队、发送消息、接收消息、撤销消息队列 d.仅当用户选择0才能结束程序的运行返回,否则继续显示功能菜单接收用户新的选择,将屏幕控制起来。
时间: 2024-02-11 10:09:14 浏览: 22
以下是实现该功能的C语言程序代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/msg.h>
#define MAX_MSG_SIZE 1024
struct msgbuf {
long mtype; // 消息类型
char mtext[MAX_MSG_SIZE]; // 消息数据
};
int main() {
int choice;
struct msgbuf msg;
int msqid;
key_t key;
int running = 1;
while (running) {
// 显示功能菜单
printf("1: get a message queue\n");
printf("2: sending message\n");
printf("3: receiving message\n");
printf("4: remove a message queue\n");
printf("0: Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
// 创建消息队列
key = ftok(".", 'a');
msqid = msgget(key, IPC_CREAT | 0666);
if (msqid == -1) {
perror("msgget");
exit(EXIT_FAILURE);
}
printf("Message queue created with ID %d.\n", msqid);
break;
case 2:
// 发送消息
printf("Enter message type: ");
scanf("%ld", &msg.mtype);
printf("Enter message data: ");
scanf("%s", msg.mtext);
msgsnd(msqid, &msg, sizeof(msg.mtext), 0);
printf("Message sent.\n");
break;
case 3:
// 接收消息
msgrcv(msqid, &msg, sizeof(msg.mtext), 0, 0);
printf("Received message type %ld: %s\n", msg.mtype, msg.mtext);
break;
case 4:
// 撤销消息队列
msgctl(msqid, IPC_RMID, NULL);
printf("Message queue removed.\n");
break;
case 0:
// 退出程序
running = 0;
break;
default:
printf("Invalid choice.\n");
break;
}
}
return 0;
}
```
该程序使用了系统提供的`msgget`、`msgsnd`、`msgrcv`、`msgctl`等函数来创建、发送、接收和撤销消息队列。用户可以根据菜单选择功能进行操作,直到选择结束程序。
相关推荐
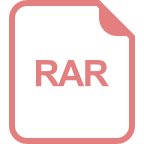
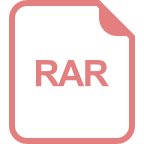











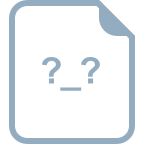
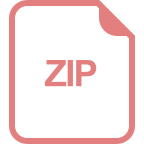
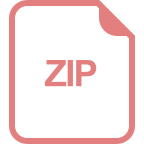