Write a C++ program that implements flipping the contents of each line of a string in a text file and outputs the flipped result to another text file. Both the input file name and output file name are specified by the user.
时间: 2024-03-01 12:50:57 浏览: 20
Sure, here's a C++ program that implements flipping the contents of each line of a string in a text file and outputs the flipped result to another text file.
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <algorithm>
using namespace std;
int main()
{
string inputFileName, outputFileName;
cout << "Enter input file name: ";
cin >> inputFileName;
cout << "Enter output file name: ";
cin >> outputFileName;
ifstream inputFile(inputFileName);
if (!inputFile.is_open())
{
cout << "Failed to open input file!" << endl;
return 1;
}
ofstream outputFile(outputFileName);
if (!outputFile.is_open())
{
cout << "Failed to open output file!" << endl;
return 1;
}
string line;
while (getline(inputFile, line))
{
reverse(line.begin(), line.end());
outputFile << line << endl;
}
inputFile.close();
outputFile.close();
cout << "File has been flipped and saved successfully!" << endl;
return 0;
}
```
The program first prompts the user to enter the input file name and output file name. It then opens the input file and output file using `ifstream` and `ofstream`, respectively. If either file cannot be opened, the program reports an error and exits.
The program then reads each line from the input file using `getline`, reverses the line using `reverse`, and writes the reversed line to the output file using `outputFile << line << endl`. Finally, the program closes both files and reports success.
Note that this program assumes that the input file contains one line of text per line, and that each line ends with a newline character. If the input file does not conform to this format, the program may not work correctly.
相关推荐
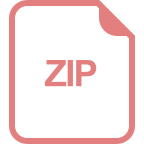














