Java使用mybatis 查询数据库表明是否存在
时间: 2023-07-21 12:31:31 浏览: 128
使用 MyBatis 查询数据库表是否存在可以分为两步:首先编写 SQL 查询语句,其次使用 MyBatis 执行 SQL 查询语句。以下是一个示例代码:
```java
import org.apache.ibatis.annotations.Param;
import org.apache.ibatis.annotations.Select;
public interface TableMapper {
@Select("SELECT COUNT(*) FROM information_schema.tables WHERE table_schema = #{dbName} AND table_name = #{tableName}")
int tableExists(@Param("dbName") String dbName, @Param("tableName") String tableName);
}
```
在这个示例中,我们使用了 `information_schema.tables` 数据库元数据表来查询表信息。如果表存在,则返回的结果是 1,否则是 0。
接下来,我们需要使用 MyBatis 执行这个 SQL 查询语句。以下是一个示例代码:
```java
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
import java.io.IOException;
import java.io.Reader;
public class CheckTableExists {
public static void main(String[] args) throws IOException {
String resource = "mybatis-config.xml";
Reader reader = Resources.getResourceAsReader(resource);
SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(reader);
SqlSession sqlSession = sqlSessionFactory.openSession();
try {
TableMapper tableMapper = sqlSession.getMapper(TableMapper.class);
int count = tableMapper.tableExists("mydatabase", "mytable");
if (count > 0) {
System.out.println("Table mytable exists.");
} else {
System.out.println("Table mytable does not exist.");
}
} finally {
sqlSession.close();
}
}
}
```
在这个示例中,我们使用 `SqlSessionFactory` 来创建一个 `SqlSession`,然后使用 `TableMapper` 来执行 SQL 查询语句。如果表存在,则输出 "Table mytable exists.",否则输出 "Table mytable does not exist."。
注意:在以上示例代码中,`mybatis-config.xml` 配置文件和 `TableMapper` 接口需要根据自己的实际情况进行修改。
阅读全文
相关推荐
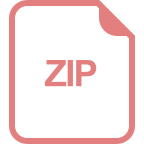
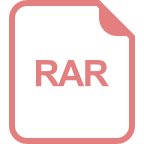
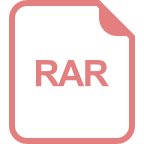
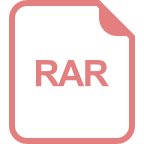
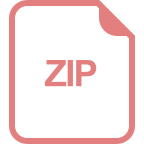
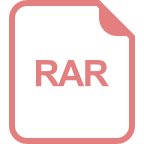
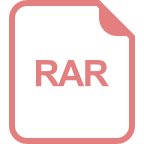
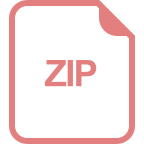
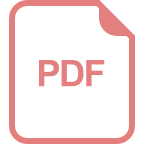
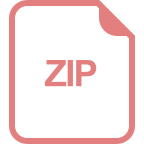
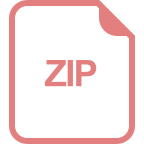
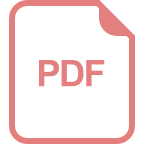
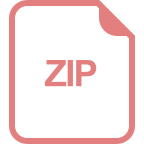
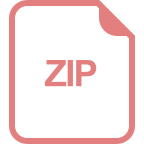
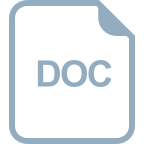
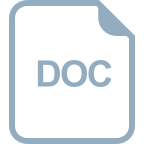