image_processor.image_mean
时间: 2024-08-15 21:05:21 浏览: 142
`image_mean`通常指的是图像处理过程中计算图像像素均值的操作。这个操作对于图像预处理、增强、分割等任务都有重要作用。
假设我们正在使用一个特定的库(如OpenCV)来进行图像处理,在Python环境下,实现计算图像均值的一种方式可能是通过遍历图像矩阵并计算其元素的平均值:
```python
from PIL import Image
# 打开源图片文件
img = Image.open('path_to_image.jpg')
# 转换为灰度图以便更容易地计算均值
gray_img = img.convert('L')
# 获取图像尺寸
width, height = gray_img.size
# 初始化像素总和与计数器
pixel_sum = 0
count = width * height
# 计算每个像素值的总和
for x in range(width):
for y in range(height):
pixel_sum += gray_img.getpixel((x, y))
# 计算像素均值
mean_pixel_value = pixel_sum / count
# 输出均值结果
print(f"Image mean pixel value: {mean_pixel_value}")
```
请注意,实际应用中可能使用的库或API会有所不同,上述代码仅作为示例。如果`image_mean`指的是某个具体库或框架中的函数,则具体的实现细节可能会有所不同。
相关问题
processor_cfg: type: "processor.pose_demo.inference" gpus: 1 worker_per_gpu: 1 video_file: resource/data_example/skateboarding.mp4 save_dir: "work_dir/pose_demo" detection_cfg: model_cfg: configs/mmdet/cascade_rcnn_r50_fpn_1x.py checkpoint_file: mmskeleton://mmdet/cascade_rcnn_r50_fpn_20e bbox_thre: 0.8 estimation_cfg: model_cfg: configs/pose_estimation/hrnet/pose_hrnet_w32_256x192_test.yaml checkpoint_file: mmskeleton://pose_estimation/pose_hrnet_w32_256x192 data_cfg: image_size: - 192 - 256 pixel_std: 200 image_mean: - 0.485 - 0.456 - 0.406 image_std: - 0.229 - 0.224 - 0.225 post_process: true argparse_cfg: gpus: bind_to: processor_cfg.gpus help: number of gpus video: bind_to: processor_cfg.video_file help: path to input video worker_per_gpu: bind_to: processor_cfg.worker_per_gpu help: number of workers for each gpu skeleton_model: bind_to: processor_cfg.estimation_cfg.model_cfg skeleton_checkpoint: bind_to: processor_cfg.estimation_cfg.checkpoint_file detection_model: bind_to: processor_cfg.detection_cfg.model_cfg detection_checkpoint: bind_to: processor_cfg.detection_cfg.checkpoint_file
根据您提供的配置文件,这是一个用于姿态估计的pose_demo的配置示例。该配置文件包括了处理器配置(processor_cfg)和命令行参数配置(argparse_cfg)。
处理器配置包括以下内容:
- type:指定处理器类型为"processor.pose_demo.inference",这可能是一个自定义的处理器类型。
- gpus:指定使用的GPU数量为1。
- worker_per_gpu:指定每个GPU的worker数量为1。
- video_file:指定输入视频的路径为"resource/data_example/skateboarding.mp4"。
- save_dir:指定结果保存的目录路径为"work_dir/pose_demo"。
检测配置(detection_cfg)包括以下内容:
- model_cfg:指定检测模型的配置文件路径为"configs/mmdet/cascade_rcnn_r50_fpn_1x.py"。
- checkpoint_file:指定检测模型的checkpoint路径为"mmskeleton://mmdet/cascade_rcnn_r50_fpn_20e"。
- bbox_thre:指定检测目标的边界框阈值为0.8。
估计配置(estimation_cfg)包括以下内容:
- model_cfg:指定姿态估计模型的配置文件路径为"configs/pose_estimation/hrnet/pose_hrnet_w32_256x192_test.yaml"。
- checkpoint_file:指定姿态估计模型的checkpoint路径为"mmskeleton://pose_estimation/pose_hrnet_w32_256x192"。
- data_cfg:指定姿态估计模型的数据配置,包括图像尺寸、像素标准化值、图像均值和标准差以及后处理选项。
命令行参数配置(argparse_cfg)包括了一些命令行参数的绑定,用于从命令行传递参数给处理器配置。
例如,您可以使用以下命令行参数来运行pose_demo:
```
python pose_demo.py --gpus 1 --video resource/data_example/skateboarding.mp4 --worker_per_gpu 1 --skeleton_model configs/pose_estimation/hrnet/pose_hrnet_w32_256x192_test.yaml --skeleton_checkpoint mmskeleton://pose_estimation/pose_hrnet_w32_256x192 --detection_model configs/mmdet/cascade_rcnn_r50_fpn_1x.py --detection_checkpoint mmskeleton://mmdet/cascade_rcnn_r50_fpn_20e
```
请注意,以上仅为示例,具体的使用方式和命令行参数可能会有所不同,具体取决于实际情况。
如果您有进一步的问题,请提供更多细节,我将尽力帮助您。
‘av_image_get_buffer_size’ was not declared in this scope; did you mean ‘av_samples_get_buffer_size’
在较新版本的 FFmpeg 库中,`av_image_get_buffer_size` 函数已被 `av_samples_get_buffer_size` 函数替代。因此,您可以将代码中的 `av_image_get_buffer_size` 替换为 `av_samples_get_buffer_size`。
以下是更新后的代码示例:
```cpp
#include <iostream>
#include <sstream>
#include <iomanip>
#include <string>
#include <chrono>
#include <ctime>
extern "C" {
#include <libavformat/avformat.h>
#include <libavcodec/avcodec.h>
}
class VideoProcessor {
public:
VideoProcessor(const std::string& rtspUrl) : rtspUrl(rtspUrl) {}
void processVideo() {
// 初始化网络
avformat_network_init();
// 打开 RTSP 流
if (avformat_open_input(&inputFormatContext, rtspUrl.c_str(), nullptr, nullptr) != 0) {
std::cerr << "无法打开视频文件" << std::endl;
return;
}
// 获取流信息
if (avformat_find_stream_info(inputFormatContext, nullptr) < 0) {
std::cerr << "无法获取视频流信息" << std::endl;
return;
}
// 输出流信息
av_dump_format(inputFormatContext, 0, rtspUrl.c_str(), 0);
// 查找视频流
AVCodecParameters* codecParameters = nullptr;
AVStream* inVStream = nullptr;
for (unsigned int i = 0; i < inputFormatContext->nb_streams; i++) {
if (inputFormatContext->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_VIDEO) {
inVStreamIndex = i;
inVStream = inputFormatContext->streams[i];
codecParameters = inputFormatContext->streams[i]->codecpar;
break;
}
}
// 检查是否找到视频流
if (inVStreamIndex == -1) {
std::cerr << "未找到视频流" << std::endl;
return;
}
// ... 其他代码逻辑 ...
}
private:
std::string rtspUrl;
AVFormatContext* inputFormatContext = nullptr;
int inVStreamIndex = -1;
};
int main() {
// RTSP地址
std::string rtspUrl = "rtsp://10.31.3.156:8554/video";
VideoProcessor videoProcessor(rtspUrl);
videoProcessor.processVideo();
return 0;
}
```
请注意,`av_samples_get_buffer_size` 函数的参数可能略有不同,具体取决于您的代码实现和需求,您可能需要根据需要进行适当的调整。
希望这可以帮助您解决问题。如果您有任何进一步的问题,请随时提问。
阅读全文
相关推荐
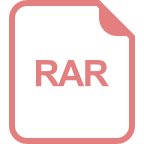
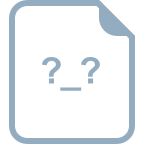
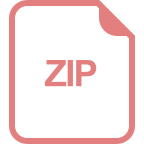
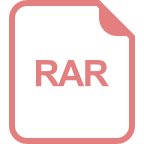
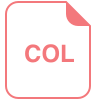
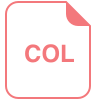
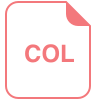
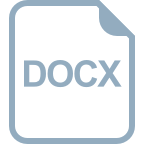
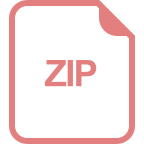