python编写Yolo识别动物图片
时间: 2023-08-16 17:03:29 浏览: 64
要使用Python编写Yolo来识别动物图片,需要以下步骤:
1. 安装Yolo及其依赖项。可以使用pip安装yolo或者从官网下载源代码并编译。
2. 下载已经训练好的Yolo模型。可以从官方网站下载或者使用其他来源。
3. 编写Python代码来使用Yolo模型进行图像识别。可以使用OpenCV库来读取和处理图像,然后使用Yolo模型来识别图像中的动物。
下面是一个简单的示例代码:
``` python
import cv2
import numpy as np
# 加载Yolo模型
net = cv2.dnn.readNet("yolov3.weights", "yolov3.cfg")
# 加载类别名称
classes = []
with open("coco.names", "r") as f:
classes = [line.strip() for line in f.readlines()]
# 读取图像
img = cv2.imread("animal.jpg")
# 调整图像大小
height, width, _ = img.shape
new_width = 416
new_height = int(height / width * new_width)
img = cv2.resize(img, (new_width, new_height))
# 将图像转换为blob
blob = cv2.dnn.blobFromImage(img, 1/255.0, (416, 416), swapRB=True, crop=False)
# 设置输入层
net.setInput(blob)
# 运行前向传播
outputs = net.forward(net.getUnconnectedOutLayersNames())
# 处理输出
boxes = []
confidences = []
class_ids = []
for output in outputs:
for detection in output:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > 0.5:
center_x = int(detection[0] * new_width)
center_y = int(detection[1] * new_height)
w = int(detection[2] * new_width)
h = int(detection[3] * new_height)
x = center_x - w // 2
y = center_y - h // 2
boxes.append([x, y, w, h])
confidences.append(float(confidence))
class_ids.append(class_id)
# 非极大值抑制
indices = cv2.dnn.NMSBoxes(boxes, confidences, 0.5, 0.4)
# 绘制边框
for i in indices:
i = i[0]
box = boxes[i]
x, y, w, h = box
label = classes[class_ids[i]]
cv2.rectangle(img, (x, y), (x+w, y+h), (0, 255, 0), 2)
cv2.putText(img, label, (x, y-10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
# 显示结果
cv2.imshow("Image", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这个例子展示了如何使用Yolo模型来识别图像中的动物,并在图像上绘制边框和标签。你需要替换其中的模型文件、类别名称和图像路径为你自己的文件路径。
相关推荐
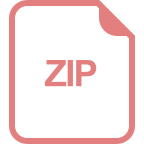














