加热炉温度控制器程序c语言
时间: 2024-01-29 19:01:08 浏览: 153
加热炉温度控制器程序的主要任务是通过控制炉内加热元件的电流来实现对温度的精确控制。以下是一个简单的C语言程序示例,用于实现加热炉温度控制器:
```c
#include <stdio.h>
// 定义炉的温度上限和下限
#define MAX_TEMP 400
#define MIN_TEMP 200
// 定义炉的加热元件电流上限和下限
#define MAX_CURRENT 10
#define MIN_CURRENT 0
int main() {
int target_temp = 300; // 设置目标温度为300
// 模拟获取当前炉温
int current_temp = 250;
// 模拟获取当前电流
int current_current = 5;
// 控制过程开始
while (1) {
// 模拟获取当前炉温
current_temp = getCurrentTemp();
// 模拟获取当前电流
current_current = getCurrentCurrent();
// 根据当前温度与目标温度的差值来调整电流
int temp_diff = target_temp - current_temp;
int current_diff = temp_diff * 0.1;
// 判断当前电流是否超过上下限
if (current_current + current_diff > MAX_CURRENT) {
current_current = MAX_CURRENT;
} else if (current_current + current_diff < MIN_CURRENT) {
current_current = MIN_CURRENT;
} else {
current_current += current_diff;
}
// 控制炉子加热元件电流
setCurrentCurrent(current_current);
// 输出当前温度和电流
printf("当前温度:%d°,当前电流:%dA\n", current_temp, current_current);
// 模拟加热炉温度变化
current_temp += (current_current - 2);
// 判断当前温度是否超过上下限
if (current_temp > MAX_TEMP) {
current_temp = MAX_TEMP;
} else if (current_temp < MIN_TEMP) {
current_temp = MIN_TEMP;
}
// 模拟设置当前温度
setCurrentTemp(current_temp);
// 休眠一段时间,等待温度变化
sleep(1);
}
return 0;
}
```
上述程序通过模拟获取当前炉温和当前电流,计算出温度与目标温度的差值,并以差值的10%作为调整电流的增量。程序使用`setCurrentCurrent(current_current)`函数来控制炉子加热元件的电流,并模拟加热炉温度的变化。程序运行时会输出当前温度和电流,并按设定的时间间隔进行循环控制加热炉温度。
阅读全文
相关推荐
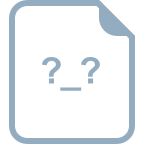
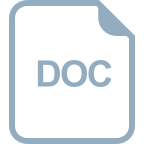
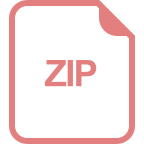
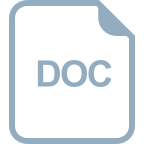
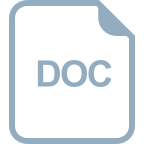
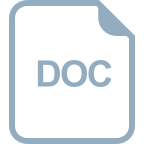
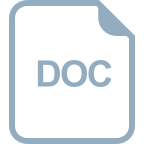
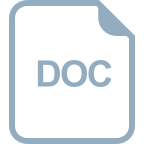
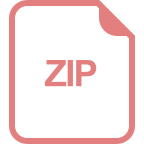
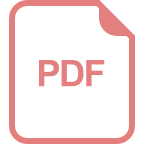
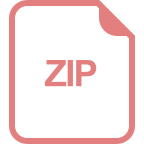
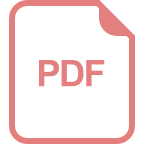
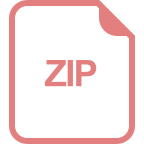
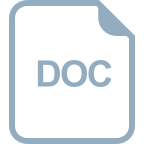
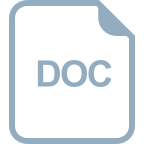
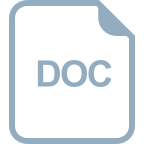