用户输入一个字符串作为密码,判断密码强度,规则为:密码长度小于8 为弱密码,密码长度大于等于8且包含至少2 种字符为中等强度;密码包含3 种字符为强;包含全部4 种字符为极强。
时间: 2024-09-23 12:02:11 浏览: 52
可以编写一个Python函数来实现这个密码强度的判断。下面是一个简单的函数,它接收一个字符串作为输入并检查其长度和字符种类:
```python
def password_strength(password):
# 定义字符种类:数字、大写字母、小写字母和其他字符
char_types = ['digit', 'upper', 'lower', 'special']
# 初始化计数器
digit_count = 0
upper_count = 0
lower_count = 0
special_count = 0
# 检查每个字符类型
for char in password:
if char.isdigit():
digit_count += 1
elif char.isupper():
upper_count += 1
elif char.islower():
lower_count += 1
else:
special_count += 1
# 判断密码强度
if len(password) < 8:
strength = "弱"
elif len(password) >= 8 and digit_count + upper_count + lower_count > 1:
strength = "中等强度" if special_count == 0 else "强"
elif len(password) >= 8 and special_count == 3:
strength = "极强"
else:
strength = "不符合规则"
return strength
# 测试示例
passwords = ["short", "8char", "has1Special", "longPasswordWithAllChars"]
for p in passwords:
print(f"{p}: {password_strength(p)}")
```
阅读全文
相关推荐
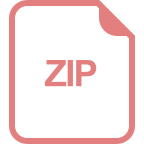
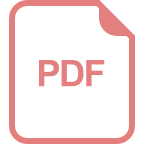
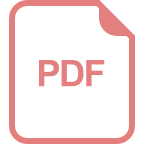













