用vue写一个页面,包含上方header栏,内容栏有六个方框,里面分别写的是xxx调查问卷模板,点击方框可跳转到相应页面,鼠标悬停到方框上,方框边缘变成淡绿色
时间: 2023-06-10 14:08:50 浏览: 102
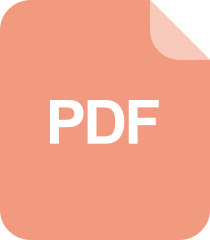
基于vue循环列表时点击跳转页面的方法
首先,我们需要安装 Vue.js,可以通过官网提供的 CDN 引入或者通过 npm 安装。
然后,我们可以按照以下步骤来实现这个页面:
1. 创建一个 Vue 实例
在 HTML 中引入 Vue.js 后,我们可以创建一个 Vue 实例,定义一个 data 对象,用来存储组件中需要用到的数据。
```html
<div id="app">
<!-- 在这里添加组件 -->
</div>
<script>
new Vue({
el: '#app',
data: {
templates: [
{ title: 'xxx调查问卷模板', link: '/template1' },
{ title: 'xxx调查问卷模板', link: '/template2' },
{ title: 'xxx调查问卷模板', link: '/template3' },
{ title: 'xxx调查问卷模板', link: '/template4' },
{ title: 'xxx调查问卷模板', link: '/template5' },
{ title: 'xxx调查问卷模板', link: '/template6' }
]
}
});
</script>
```
在 data 对象中,我们定义了一个名为 templates 的数组,用来存储六个方框的标题和链接地址。
2. 创建 Header 组件
```html
<template>
<header>
<h1>Header</h1>
</header>
</template>
```
这里我们只是简单地创建了一个 header 组件,并在其中添加了一个 h1 标签。
3. 创建 Template 组件
```html
<template>
<div class="template" v-for="(template, index) in templates" :key="index" :style="{ backgroundImage: `url(${template.image})` }">
<a :href="template.link" @mouseover="hover = true" @mouseleave="hover = false">
<h2>{{ template.title }}</h2>
</a>
</div>
</template>
<script>
export default {
data() {
return {
hover: false
}
}
}
</script>
```
这里我们创建了一个名为 Template 的组件,使用了 v-for 指令来循环遍历 templates 数组,然后通过绑定样式的方式,将每个方框的背景图片设为 templates 数组中对应的图片。
在每个方框中,我们添加了一个 a 标签,用来实现点击跳转到相应页面的功能,并且通过绑定 @mouseover 和 @mouseleave 事件,实现鼠标悬停时方框边缘变成淡绿色的效果。
4. 组合组件
将 Header 和 Template 组件组合起来,就可以得到最终的页面了。
```html
<template>
<div>
<Header />
<div class="content">
<Template />
</div>
</div>
</template>
<script>
import Header from './components/Header.vue';
import Template from './components/Template.vue';
export default {
components: {
Header,
Template
}
}
</script>
<style>
.content {
display: flex;
flex-wrap: wrap;
justify-content: space-between;
max-width: 1000px;
margin: 0 auto;
padding: 50px 0;
}
.template {
width: calc(33.33% - 20px);
height: 200px;
margin-bottom: 40px;
background-size: cover;
background-position: center center;
border: 1px solid #ccc;
border-radius: 5px;
transition: border-color 0.3s ease;
display: flex;
justify-content: center;
align-items: center;
}
.template:hover {
border-color: #b2dfdb;
}
h2 {
font-size: 24px;
color: #fff;
text-shadow: 1px 1px 1px rgba(0, 0, 0, 0.5);
text-align: center;
}
a {
display: block;
width: 100%;
height: 100%;
}
</style>
```
在组合组件的时候,我们引入了 Header 和 Template 组件,并在 components 属性中注册了它们。
在样式中,我们对 content 和 template 进行了一些布局和样式的设置,实现了页面的效果。
阅读全文
相关推荐
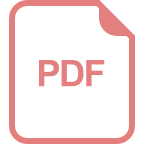
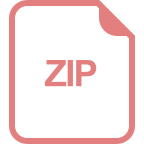
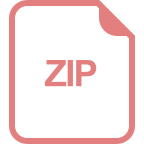
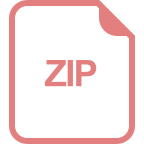
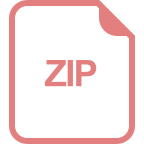
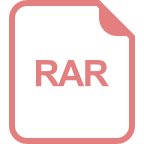
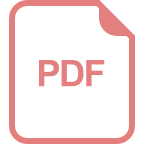
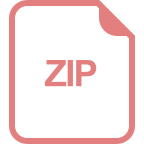
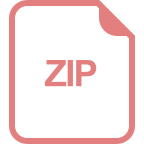
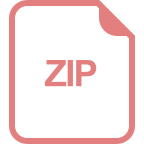
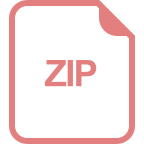
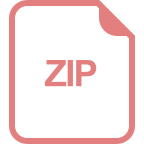
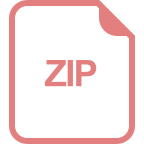
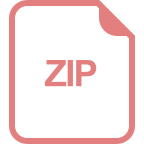
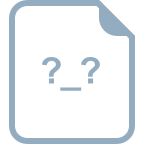
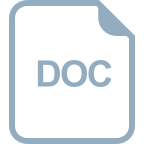

