python数据库增删改查
时间: 2023-07-25 15:43:16 浏览: 53
好的,下面是Python数据库增删改查的示例代码:
#### 连接数据库
```python
import sqlite3
conn = sqlite3.connect('example.db')
```
#### 创建表
```python
c = conn.cursor()
# 创建一个名为students的表,包含id, name, age和gender四个字段
c.execute('''CREATE TABLE students
(id INT PRIMARY KEY NOT NULL,
name TEXT NOT NULL,
age INT NOT NULL,
gender CHAR(10) NOT NULL);''')
```
#### 插入数据
```python
# 插入一条记录
c.execute("INSERT INTO students (id, name, age, gender) VALUES (1, 'Tom', 18, 'Male');")
# 插入多条记录
students = [(2, 'Jerry', 17, 'Female'), (3, 'Bob', 19, 'Male')]
c.executemany("INSERT INTO students (id, name, age, gender) VALUES (?, ?, ?, ?);", students)
# 提交事务
conn.commit()
```
#### 查询数据
```python
# 查询所有记录
c.execute("SELECT * FROM students;")
rows = c.fetchall()
for row in rows:
print(row)
# 查询年龄小于20岁的记录
c.execute("SELECT * FROM students WHERE age < 20;")
rows = c.fetchall()
for row in rows:
print(row)
```
#### 更新数据
```python
# 将id为1的记录的年龄改为20岁
c.execute("UPDATE students SET age = 20 WHERE id = 1;")
# 提交事务
conn.commit()
```
#### 删除数据
```python
# 删除id为2的记录
c.execute("DELETE FROM students WHERE id = 2;")
# 提交事务
conn.commit()
```
#### 关闭连接
```python
conn.close()
```
以上是SQLite数据库的示例代码,不同的数据库类型可能有些许差异,但基本思路是相同的。
相关推荐
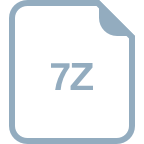














