图片转字符画c++
时间: 2023-08-05 22:06:31 浏览: 160
实现图片转字符画的 C++ 代码如下:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <opencv2/opencv.hpp>
using namespace std;
using namespace cv;
// 将像素点转换为字符画
char pixelToChar(int pixel) {
// 灰度值映射为 ASCII 字符
const char ascii[] = {' ', '.', '\'', ':', 'o', '&', '8', '#', '@'};
int gray = (int)(0.2126 * ((pixel & 0xff0000) >> 16) + 0.7152 * ((pixel & 0x00ff00) >> 8) + 0.0722 * (pixel & 0x0000ff));
int index = (gray * 9) / 255;
return ascii[index];
}
int main(int argc, char **argv) {
// 读取图片
Mat img = imread(argv[1]);
if (img.empty()) {
cout << "Could not open or find the image" << endl;
return -1;
}
// 图片尺寸缩小
Mat resizedImg;
resize(img, resizedImg, Size(), 0.2, 0.2, INTER_AREA);
// 打开输出文件
ofstream fout("output.txt");
// 将像素点转换为字符画,并输出到文件
for (int i = 0; i < resizedImg.rows; i++) {
for (int j = 0; j < resizedImg.cols; j++) {
fout << pixelToChar(resizedImg.at<Vec3b>(i, j)[0] << 16 | resizedImg.at<Vec3b>(i, j)[1] << 8 | resizedImg.at<Vec3b>(i, j)[2]);
}
fout << endl;
}
// 关闭输出文件
fout.close();
return 0;
}
```
代码中使用 OpenCV 库读取图片,将图片缩小到原大小的 20% ,并将每个像素点转换为字符画,输出到文件中。转换过程中使用灰度值映射为 ASCII 字符,最终输出的字符画效果取决于映射表的选择。
阅读全文
相关推荐
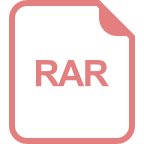
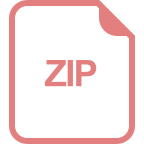
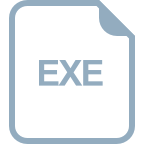
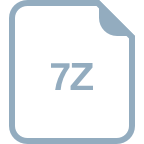
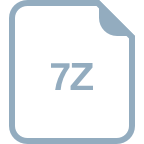
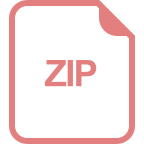
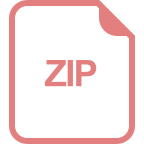
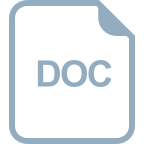
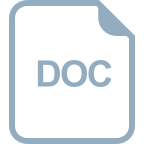
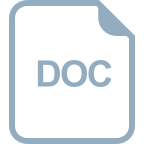

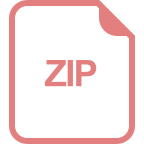
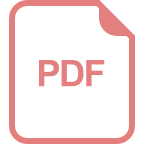
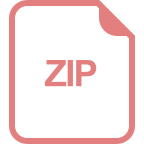
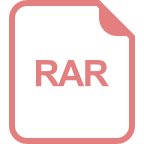
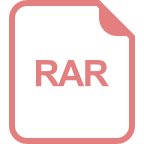
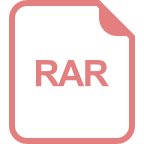
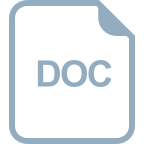