c++17 复制共享文件夹中的图像、
时间: 2024-01-28 12:04:08 浏览: 31
要复制共享文件夹中的图像,您可以使用C++17的`std::filesystem`库,具体步骤如下:
1. 连接到共享文件夹:您需要使用SMB客户端或其他工具将共享文件夹挂载到本地文件系统。
2. 构造目标文件夹路径:您需要指定一个本地文件夹作为目标文件夹,并使用`std::filesystem::path`类构造目标文件夹路径。
3. 遍历共享文件夹中的图像文件:您可以使用`std::filesystem::recursive_directory_iterator`类遍历共享文件夹中的所有文件,然后对每个文件进行判断,如果是图像文件,就将其复制到目标文件夹中。
下面是一个使用C++17实现此操作的示例代码:
```c++
#include <iostream>
#include <filesystem>
int main()
{
// 连接到共享文件夹,将共享文件夹挂载到本地文件系统
std::filesystem::path shared_folder_path = "/mnt/shared_folder";
// 构造目标文件夹路径
std::filesystem::path target_folder_path = "./resultImage";
// 遍历共享文件夹中的图像文件
for (auto& file : std::filesystem::recursive_directory_iterator(shared_folder_path))
{
// 判断文件是否为图像文件
if (file.is_regular_file() && file.path().extension() == ".jpg")
{
// 构造目标文件路径
std::filesystem::path target_file_path = target_folder_path / file.path().filename();
try
{
// 复制文件
std::filesystem::copy_file(file.path(), target_file_path, std::filesystem::copy_options::update_existing);
std::cout << "File copied successfully: " << file.path() << std::endl;
}
catch (const std::exception& e)
{
std::cerr << "Error: " << e.what() << std::endl;
}
}
}
return 0;
}
```
在这个示例中,我们首先构造了共享文件夹路径和目标文件夹路径,然后使用`std::filesystem::recursive_directory_iterator`遍历共享文件夹中的所有文件,对于每个文件,我们判断其是否为图像文件,如果是,则构造目标文件路径,并使用`std::filesystem::copy_file`函数将其复制到目标文件夹中。在复制过程中,如果发生异常,我们将捕获并打印异常信息。
相关推荐
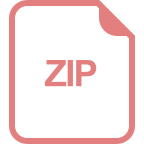
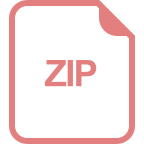
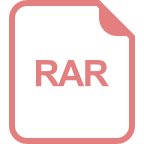














