Please define a class DoubleValue that wraps(包装) a value of primitive type double and satisfies the following requirements: (1) it has a default constructor which sets the value to 0.0; (2) it has a constructor with one argument of type double that is wrapped; (3) by overloading the operator “==”, it can compare this object against another specified DoubleValue object, and return true if and only if both DoubleValue represent the same double value; (4) it can return a string representation of the wrapped double value; (5) it can return the value of this DoubleValue as an int type after a narrowing primitive conversion.
时间: 2024-02-14 10:18:37 浏览: 94
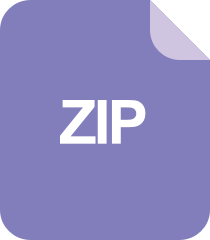
C Primer Plus 附录A 复习题答案
Sure, here's a possible implementation of the DoubleValue class in C++:
```cpp
#include <string>
class DoubleValue {
public:
DoubleValue() : value(0.0) {}
DoubleValue(double v) : value(v) {}
bool operator==(const DoubleValue& other) const {
return value == other.value;
}
std::string to_string() const {
return std::to_string(value);
}
int to_int() const {
return static_cast<int>(value);
}
private:
double value;
};
```
The class has two constructors, as required: a default constructor that sets the value to 0.0, and a constructor with one argument of type double that sets the wrapped value to the specified value.
The `operator==` is overloaded to compare two DoubleValue objects by their wrapped values, returning true if and only if they are equal.
The `to_string` method returns a string representation of the wrapped double value, using the `std::to_string` function from the C++ standard library.
The `to_int` method returns the value of the DoubleValue as an int type after a narrowing primitive conversion, using the `static_cast` operator.
Hope this helps!
阅读全文
相关推荐
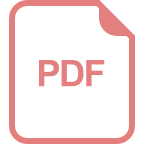
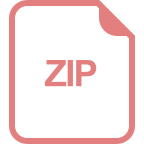
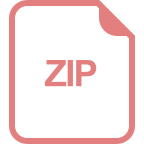
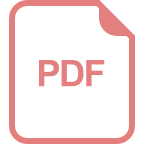
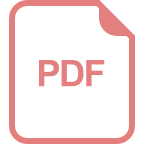
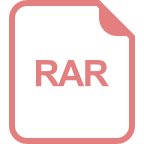
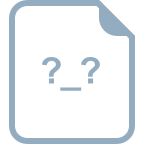
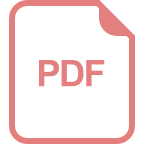
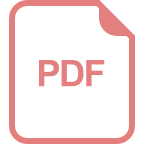
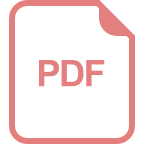
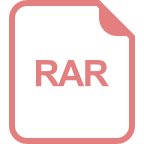
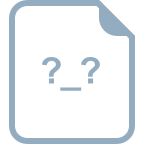
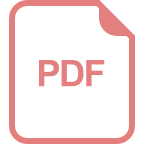
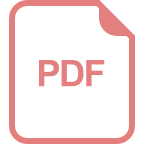
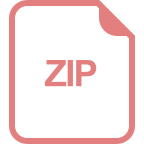